Prune Dataset to extract representative subset#
In this notebook example, we will demonstrate the process of creating a pruned dataset, which is a representative subset of the original dataset. By working with a smaller, yet representative, subset of the training data, we can observe how the model’s performance is affected in terms of accuracy and convergence speed. Through this, we can check accuracy drop and convergence time with subset of training data through OpenVINO™ Training Extensions. This analysis provides valuable insights into the relationship between dataset size and model performance. It helps us understand the scalability and generalization capabilities of the model, shedding light on the efficiency of training algorithms and the potential benefits of working with a pruned dataset. It also allows us to assess the impact of dataset size on model performance, providing guidance for resource allocation and model development in practical scenarios.
Prerequisite#
Download Caltech-101 dataset#
This is a download link for caltech101 dataset in Kaggle. Please download using this link and extract to your workspace directory. Then, you will have a caltech-101
directory with images in imagenet format as follows.
caltech-101
├── accordion
│ ├── image_0001.jpg
│ ├── image_0002.jpg
│ ├── ...
├── airplanes
│ ├── image_0001.jpg
│ ├── image_0002.jpg
│ ├── ...
│ ...
└── yin_yang
├── image_0001.jpg
├── image_0002.jpg
├── ...
Install OpenVINO™ Training Extensions#
For more details, please see this OpenVINO™ Training Extensions installation guide.
[ ]:
!pip install otx
Prune dataset using Datumaro Python API#
In this section, we utilize the Dataumaro Python API to prune entire dataset. We import the caltech-101 dataset and apply dataset prune.
[1]:
import datumaro as dm
from datumaro.components.algorithms.hash_key_inference.prune import Prune
dataset = dm.Dataset.import_from("caltech-101", format="imagenet")
dataset
2023-07-08 01:40:37.166610: I tensorflow/core/platform/cpu_feature_guard.cc:182] This TensorFlow binary is optimized to use available CPU instructions in performance-critical operations.
To enable the following instructions: AVX2 FMA, in other operations, rebuild TensorFlow with the appropriate compiler flags.
2023-07-08 01:40:37.725001: W tensorflow/compiler/tf2tensorrt/utils/py_utils.cc:38] TF-TRT Warning: Could not find TensorRT
[1]:
Dataset
size=9144
source_path=caltech-101
media_type=<class 'datumaro.components.media.Image'>
annotated_items_count=9144
annotations_count=9144
subsets
default: # of items=9144, # of annotated items=9144, # of annotations=9144, annotation types=['label']
infos
categories
label: ['BACKGROUND_Google', 'Faces', 'Faces_easy', 'Leopards', 'Motorbikes', 'accordion', 'airplanes', 'anchor', 'ant', 'barrel', 'bass', 'beaver', 'binocular', 'bonsai', 'brain', 'brontosaurus', 'buddha', 'butterfly', 'camera', 'cannon', 'car_side', 'ceiling_fan', 'cellphone', 'chair', 'chandelier', 'cougar_body', 'cougar_face', 'crab', 'crayfish', 'crocodile', 'crocodile_head', 'cup', 'dalmatian', 'dollar_bill', 'dolphin', 'dragonfly', 'electric_guitar', 'elephant', 'emu', 'euphonium', 'ewer', 'ferry', 'flamingo', 'flamingo_head', 'garfield', 'gerenuk', 'gramophone', 'grand_piano', 'hawksbill', 'headphone', 'hedgehog', 'helicopter', 'ibis', 'inline_skate', 'joshua_tree', 'kangaroo', 'ketch', 'lamp', 'laptop', 'llama', 'lobster', 'lotus', 'mandolin', 'mayfly', 'menorah', 'metronome', 'minaret', 'nautilus', 'octopus', 'okapi', 'pagoda', 'panda', 'pigeon', 'pizza', 'platypus', 'pyramid', 'revolver', 'rhino', 'rooster', 'saxophone', 'schooner', 'scissors', 'scorpion', 'sea_horse', 'snoopy', 'soccer_ball', 'stapler', 'starfish', 'stegosaurus', 'stop_sign', 'strawberry', 'sunflower', 'tick', 'trilobite', 'umbrella', 'watch', 'water_lilly', 'wheelchair', 'wild_cat', 'windsor_chair', 'wrench', 'yin_yang']
Generate the validation report#
Before pruning entire dataset, we first generate the validation report of dataset to confirm the statistics of dataset labels.
[2]:
from datumaro.plugins.validators import ClassificationValidator
from matplotlib import pyplot as plt
validator = ClassificationValidator()
reports = validator.validate(dataset)
The label distribution in the entire dataset is as follows.
[3]:
stats = reports["statistics"]
label_stats = stats["label_distribution"]["defined_labels"]
label_name, label_counts = zip(*[(k, v) for k, v in label_stats.items()])
plt.figure(figsize=(12, 4))
plt.hist(label_name, weights=label_counts, bins=len(label_name))
plt.xticks(rotation="vertical")
plt.show()
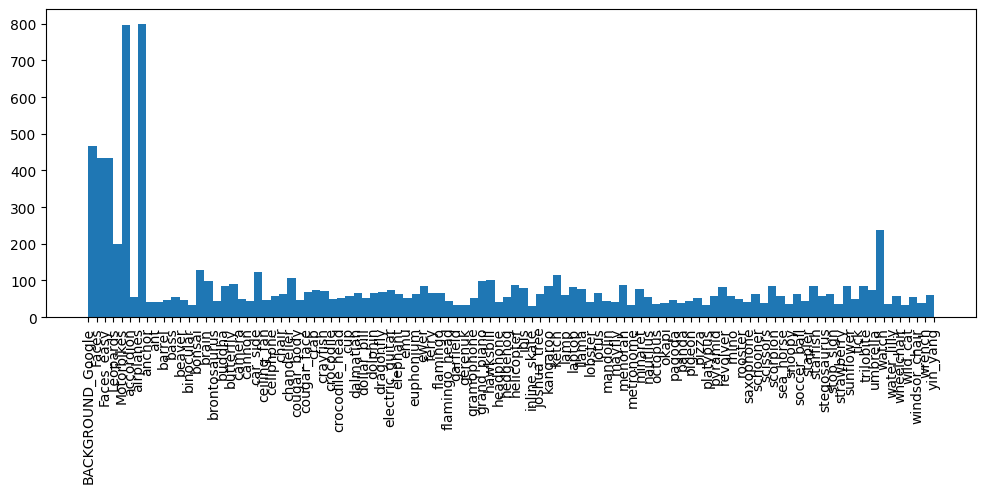
Prune dataset#
We support various prune methods in Datumaro. We will try each method and compare the results by examining the train and validation reports. We will start by checking using the random
method. For all methods, we will use a ratio of 0.5
.
The random method involves randomly selecting dataset items from the entire dataset to create a subset.
[4]:
prune = Prune(dataset, cluster_method="random")
random_result = prune.get_pruned(0.5)
When creating a subset using the random method, as shown below, we can observe that the label distribution changes.
[5]:
random_reports = validator.validate(random_result)
random_stats = random_reports["statistics"]
label_stats = random_stats["label_distribution"]["defined_labels"]
label_name, label_counts = zip(*[(k, v) for k, v in label_stats.items()])
plt.figure(figsize=(12, 4))
plt.hist(label_name, weights=label_counts, bins=len(label_name))
plt.xticks(rotation="vertical")
plt.show()
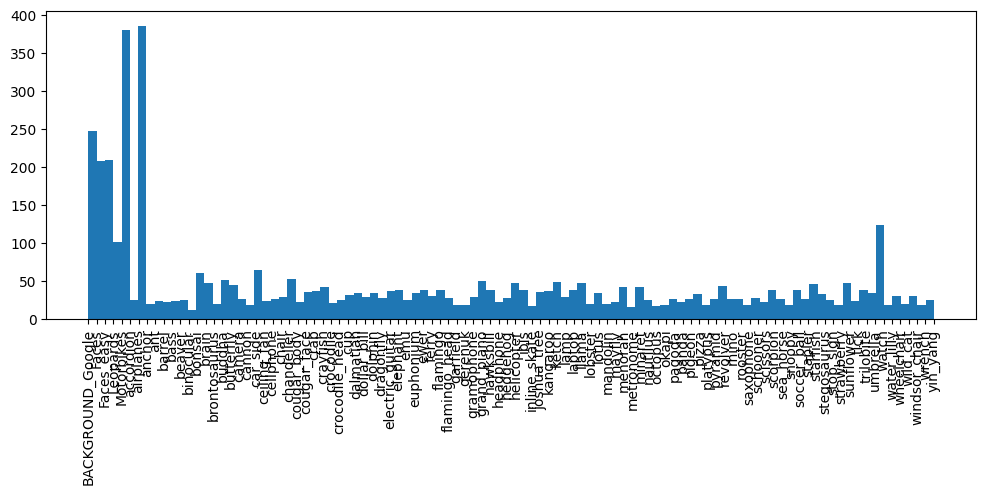
In this case, we use cluster_random
method. For detail information about each method, please refer prune.
[6]:
prune = Prune(dataset, cluster_method="cluster_random")
cluster_random_result = prune.get_pruned(0.5)
When creating a subset using the cluster random method, as shown below, we can observe that the label distribution changes. In the case of this dataset, the distribution of each class did not change significantly.
[7]:
cluster_random_reports = validator.validate(cluster_random_result)
cluster_random_stats = cluster_random_reports["statistics"]
label_stats = cluster_random_stats["label_distribution"]["defined_labels"]
label_name, label_counts = zip(*[(k, v) for k, v in label_stats.items()])
plt.figure(figsize=(12, 4))
plt.hist(label_name, weights=label_counts, bins=len(label_name))
plt.xticks(rotation="vertical")
plt.show()
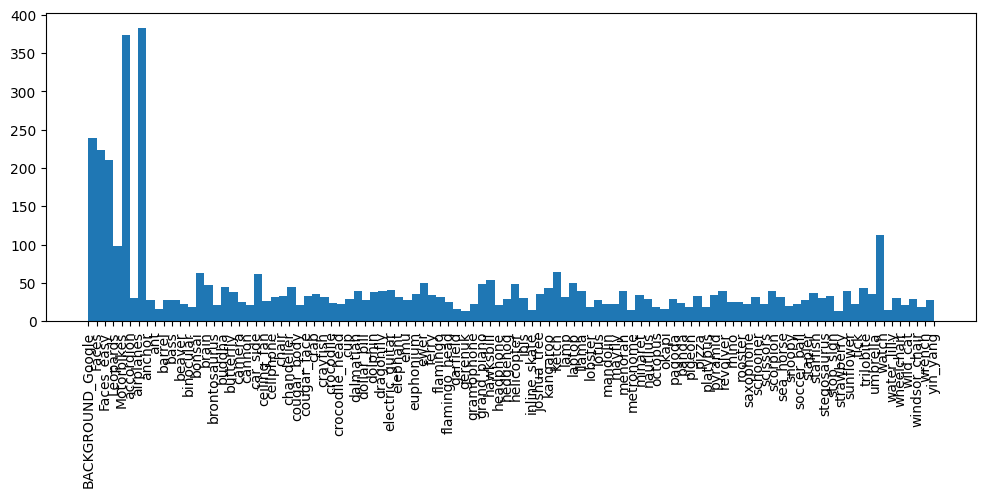
We use query_clust
method. For detail information about each method, please refer prune.
[8]:
prune = Prune(dataset, cluster_method="query_clust")
query_clust_result = prune.get_pruned(0.5)
When creating a subset using the query clust method, as shown below, we can observe that the label distribution changes. In the caltech-101 dataset, when the datasets included in each class were small, there is a tendency for the ratio of classes with more data to increase compared to the datasets of those classes. This tendency may vary for different datasets, so we recommend comparing different methods directly on your own data.
[9]:
query_clust_reports = validator.validate(query_clust_result)
query_clust_stats = query_clust_reports["statistics"]
label_stats = query_clust_stats["label_distribution"]["defined_labels"]
label_name, label_counts = zip(*[(k, v) for k, v in label_stats.items()])
plt.figure(figsize=(12, 4))
plt.hist(label_name, weights=label_counts, bins=len(label_name))
plt.xticks(rotation="vertical")
plt.show()
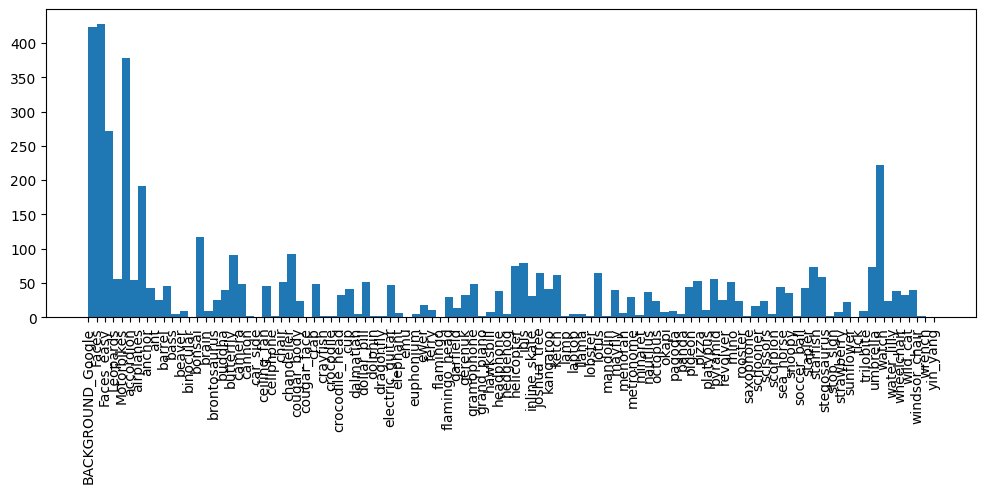
We use centroid
method. For detail information about each method, please refer prune.
[10]:
prune = Prune(dataset, cluster_method="centroid")
centroid_result = prune.get_pruned(0.5)
When creating a subset using the centroid method, as shown below, we can observe that the label distribution changes. In this case, we can see that the proportion of motorbike, which had a large amount of dataset, has decreased. This illustrates the tendency of certain classes to have a reduced proportion of their data within the overall dataset. It is possible to identify such trends where the contribution of data from specific classes decreases within the entire dataset.
[11]:
centroid_reports = validator.validate(centroid_result)
centroid_stats = centroid_reports["statistics"]
label_stats = centroid_stats["label_distribution"]["defined_labels"]
label_name, label_counts = zip(*[(k, v) for k, v in label_stats.items()])
plt.figure(figsize=(12, 4))
plt.hist(label_name, weights=label_counts, bins=len(label_name))
plt.xticks(rotation="vertical")
plt.show()
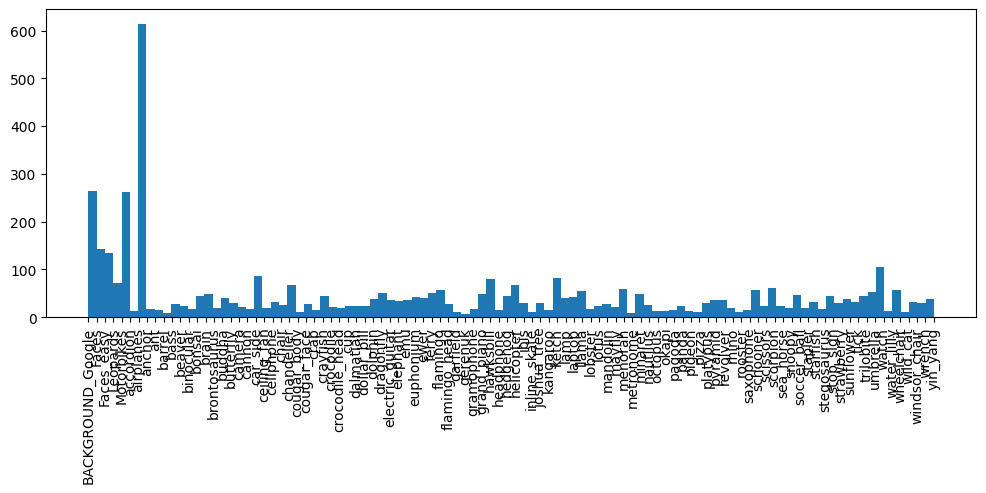
We use entropy
method. For detail information about each method, please refer prune.
[12]:
prune = Prune(dataset, cluster_method="entropy")
entropy_result = prune.get_pruned(0.5)
When creating a subset using the entropy method, as shown below, we can observe that the label distribution changes.
[13]:
entropy_reports = validator.validate(entropy_result)
entropy_stats = entropy_reports["statistics"]
label_stats = entropy_stats["label_distribution"]["defined_labels"]
label_name, label_counts = zip(*[(k, v) for k, v in label_stats.items()])
plt.figure(figsize=(12, 4))
plt.hist(label_name, weights=label_counts, bins=len(label_name))
plt.xticks(rotation="vertical")
plt.show()
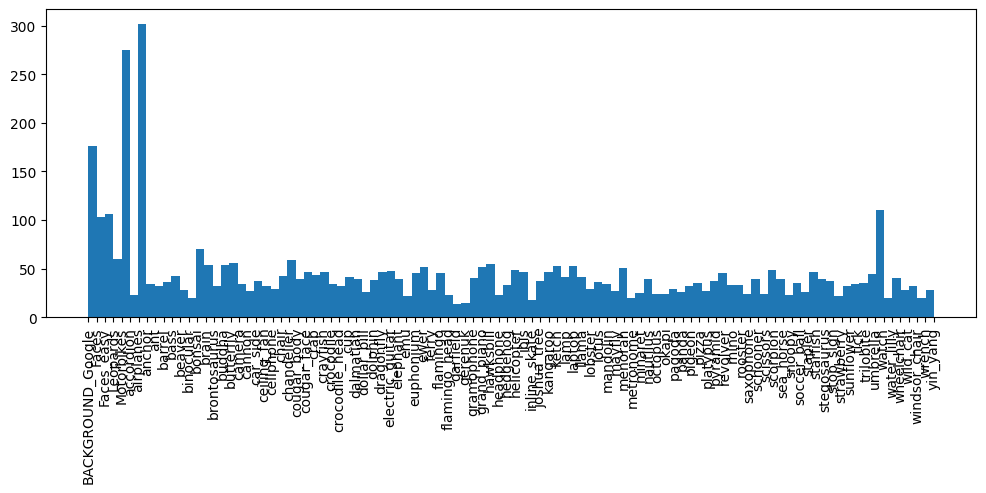
Finally, we use ndr
method. For detail information about each method, please refer prune.
[14]:
prune = Prune(dataset, cluster_method="ndr")
ndr_result = prune.get_pruned(0.5)
When creating a subset using the ndr method, as shown below, we can observe that the label distribution changes.
[15]:
ndr_reports = validator.validate(ndr_result)
ndr_stats = ndr_reports["statistics"]
label_stats = ndr_stats["label_distribution"]["defined_labels"]
label_name, label_counts = zip(*[(k, v) for k, v in label_stats.items()])
plt.figure(figsize=(12, 4))
plt.hist(label_name, weights=label_counts, bins=len(label_name))
plt.xticks(rotation="vertical")
plt.show()
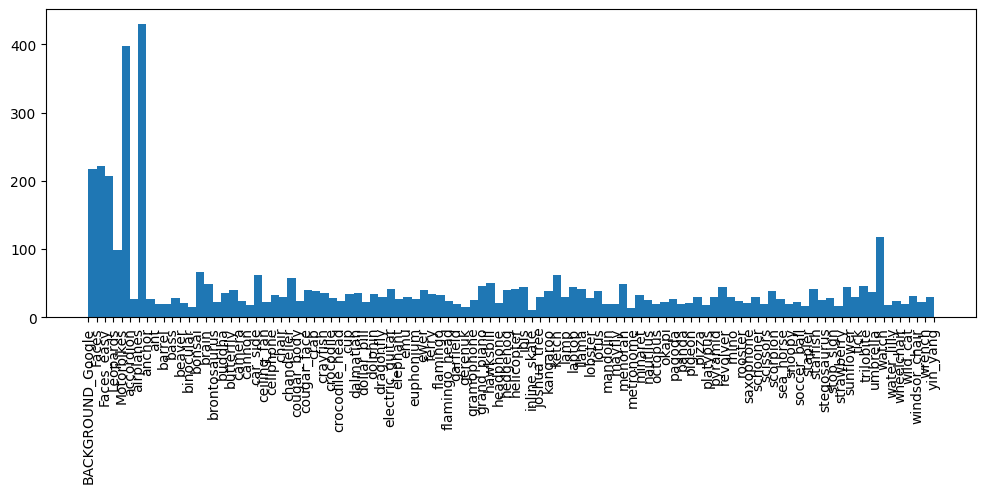
We export those pruned subset to train the model with OpenVINO™ Training Extensions.
[16]:
random_result.export("random_result", format="datumaro", save_media=True)
cluster_random_result.export("cluster_random_result", format="datumaro", save_media=True)
query_clust_result.export("query_clust_result", format="datumaro", save_media=True)
centroid_result.export("centroid_result", format="datumaro", save_media=True)
entropy_result.export("entropy_result", format="datumaro", save_media=True)
ndr_result.export("ndr_result", format="datumaro", save_media=True)
Train Model and Export the Trained Classification Model Using OpenVINO™ Training Extensions#
In this step, we train a classification model using OTX. To see the detail guides for OpenVINO™ Training Extensions usage, please see How-To-Train. In this example, we use the CLI command to train the model and choose EfficientNet-B0
model supported by OpenVINO™ Training Extensions.
[1]:
!otx train EfficientNet-B0 \
--train-data-roots caltech-101 \
--val-data-roots caltech-101 \
-o outputs
[*] Workspace Path: otx-workspace-CLASSIFICATION
[*] Load Model Template ID: Custom_Image_Classification_EfficinetNet-B0
[*] Load Model Name: EfficientNet-B0
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/mmcv/__init__.py:20: UserWarning: On January 1, 2023, MMCV will release v2.0.0, in which it will remove components related to the training process and add a data transformation module. In addition, it will rename the package names mmcv to mmcv-lite and mmcv-full to mmcv. See https://github.com/open-mmlab/mmcv/blob/master/docs/en/compatibility.md for more details.
warnings.warn(
2023-07-10 18:25:50,278 | WARNING : Duplicate key is detected among bases [{'model'}]
[*] - Updated: otx-workspace-CLASSIFICATION/model.py
2023-07-10 18:25:50,307 | WARNING : Duplicate key is detected among bases [{'model'}]
[*] - Updated: otx-workspace-CLASSIFICATION/model_multilabel.py
[*] - Updated: otx-workspace-CLASSIFICATION/data_pipeline.py
[*] - Updated: otx-workspace-CLASSIFICATION/deployment.py
[*] - Updated: otx-workspace-CLASSIFICATION/hpo_config.yaml
2023-07-10 18:25:50,407 | WARNING : Duplicate key is detected among bases [{'model'}]
[*] - Updated: otx-workspace-CLASSIFICATION/model_hierarchical.py
[*] - Updated: otx-workspace-CLASSIFICATION/compression_config.json
[*] Update data configuration file to: otx-workspace-CLASSIFICATION/data.yaml
2023-07-10 18:25:50.679934: I tensorflow/core/platform/cpu_feature_guard.cc:182] This TensorFlow binary is optimized to use available CPU instructions in performance-critical operations.
To enable the following instructions: AVX2 FMA, in other operations, rebuild TensorFlow with the appropriate compiler flags.
2023-07-10 18:25:51.257037: W tensorflow/compiler/tf2tensorrt/utils/py_utils.cc:38] TF-TRT Warning: Could not find TensorRT
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/openvino/pyopenvino/__init__.py:10: FutureWarning: The module is private and following namespace `pyopenvino` will be removed in the future
warnings.warn(message="The module is private and following namespace " "`pyopenvino` will be removed in the future", category=FutureWarning)
2023-07-10 18:25:54,091 | INFO : Classification mode: multiclass
2023-07-10 18:25:54,091 | INFO : train()
2023-07-10 18:25:54,104 | INFO : Training seed was set to 5 w/ deterministic=False.
2023-07-10 18:25:54,106 | INFO : Try to create a 0 size memory pool.
2023-07-10 18:25:55,390 | INFO : configure!: training=True
2023-07-10 18:25:55,504 | INFO : init weight - https://github.com/osmr/imgclsmob/releases/download/v0.0.364/efficientnet_b0-0752-0e386130.pth.zip
2023-07-10 18:25:55,527 | INFO : 'in_channels' config in model.head is updated from -1 to 1280
2023-07-10 18:25:55,528 | INFO : configure_data()
2023-07-10 18:25:55,528 | INFO : task config!!!!: training=True
2023-07-10 18:25:55,528 | INFO : train!
2023-07-10 18:25:55,528 | INFO : cfg.gpu_ids = range(0, 1), distributed = False
2023-07-10 18:25:55,547 | INFO : Environment info:
------------------------------------------------------------
sys.platform: linux
Python: 3.10.0 (default, Mar 3 2022, 09:58:08) [GCC 7.5.0]
CUDA available: True
GPU 0,1: GeForce RTX 3090
CUDA_HOME: /usr/local/cuda
NVCC: Cuda compilation tools, release 11.1, V11.1.74
GCC: gcc (Ubuntu 7.5.0-3ubuntu1~18.04) 7.5.0
PyTorch: 1.13.1+cu117
PyTorch compiling details: PyTorch built with:
- GCC 9.3
- C++ Version: 201402
- Intel(R) Math Kernel Library Version 2020.0.0 Product Build 20191122 for Intel(R) 64 architecture applications
- Intel(R) MKL-DNN v2.6.0 (Git Hash 52b5f107dd9cf10910aaa19cb47f3abf9b349815)
- OpenMP 201511 (a.k.a. OpenMP 4.5)
- LAPACK is enabled (usually provided by MKL)
- NNPACK is enabled
- CPU capability usage: AVX2
- CUDA Runtime 11.7
- NVCC architecture flags: -gencode;arch=compute_37,code=sm_37;-gencode;arch=compute_50,code=sm_50;-gencode;arch=compute_60,code=sm_60;-gencode;arch=compute_70,code=sm_70;-gencode;arch=compute_75,code=sm_75;-gencode;arch=compute_80,code=sm_80;-gencode;arch=compute_86,code=sm_86
- CuDNN 8.5
- Magma 2.6.1
- Build settings: BLAS_INFO=mkl, BUILD_TYPE=Release, CUDA_VERSION=11.7, CUDNN_VERSION=8.5.0, CXX_COMPILER=/opt/rh/devtoolset-9/root/usr/bin/c++, CXX_FLAGS= -fabi-version=11 -Wno-deprecated -fvisibility-inlines-hidden -DUSE_PTHREADPOOL -fopenmp -DNDEBUG -DUSE_KINETO -DUSE_FBGEMM -DUSE_QNNPACK -DUSE_PYTORCH_QNNPACK -DUSE_XNNPACK -DSYMBOLICATE_MOBILE_DEBUG_HANDLE -DEDGE_PROFILER_USE_KINETO -O2 -fPIC -Wno-narrowing -Wall -Wextra -Werror=return-type -Werror=non-virtual-dtor -Wno-missing-field-initializers -Wno-type-limits -Wno-array-bounds -Wno-unknown-pragmas -Wunused-local-typedefs -Wno-unused-parameter -Wno-unused-function -Wno-unused-result -Wno-strict-overflow -Wno-strict-aliasing -Wno-error=deprecated-declarations -Wno-stringop-overflow -Wno-psabi -Wno-error=pedantic -Wno-error=redundant-decls -Wno-error=old-style-cast -fdiagnostics-color=always -faligned-new -Wno-unused-but-set-variable -Wno-maybe-uninitialized -fno-math-errno -fno-trapping-math -Werror=format -Werror=cast-function-type -Wno-stringop-overflow, LAPACK_INFO=mkl, PERF_WITH_AVX=1, PERF_WITH_AVX2=1, PERF_WITH_AVX512=1, TORCH_VERSION=1.13.1, USE_CUDA=ON, USE_CUDNN=ON, USE_EXCEPTION_PTR=1, USE_GFLAGS=OFF, USE_GLOG=OFF, USE_MKL=ON, USE_MKLDNN=ON, USE_MPI=OFF, USE_NCCL=ON, USE_NNPACK=ON, USE_OPENMP=ON, USE_ROCM=OFF,
TorchVision: 0.14.1+cu117
OpenCV: 4.7.0
MMCV: 1.7.1
MMCV Compiler: GCC 7.5
MMCV CUDA Compiler: 11.1
MMClassification: 0.25.0+c5ac764
------------------------------------------------------------
2023-07-10 18:25:55,910 | INFO : init weight - https://github.com/osmr/imgclsmob/releases/download/v0.0.364/efficientnet_b0-0752-0e386130.pth.zip
2023-07-10 18:25:55,919 - mmcv - INFO - initialize CustomLinearClsHead with init_cfg {'type': 'Normal', 'layer': 'Linear', 'std': 0.01}
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.init_block.conv.conv.weight - torch.Size([32, 3, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.init_block.conv.bn.weight - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.init_block.conv.bn.bias - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage1.unit1.dw_conv.conv.weight - torch.Size([32, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage1.unit1.dw_conv.bn.weight - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage1.unit1.dw_conv.bn.bias - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv1.weight - torch.Size([8, 32, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv1.bias - torch.Size([8]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv2.weight - torch.Size([32, 8, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv2.bias - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage1.unit1.pw_conv.conv.weight - torch.Size([16, 32, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage1.unit1.pw_conv.bn.weight - torch.Size([16]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage1.unit1.pw_conv.bn.bias - torch.Size([16]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage2.unit1.conv1.conv.weight - torch.Size([96, 16, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage2.unit1.conv1.bn.weight - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage2.unit1.conv1.bn.bias - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage2.unit1.conv2.conv.weight - torch.Size([96, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage2.unit1.conv2.bn.weight - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage2.unit1.conv2.bn.bias - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv1.weight - torch.Size([4, 96, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,920 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv1.bias - torch.Size([4]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv2.weight - torch.Size([96, 4, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv2.bias - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit1.conv3.conv.weight - torch.Size([24, 96, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit1.conv3.bn.weight - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit1.conv3.bn.bias - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.conv1.conv.weight - torch.Size([144, 24, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.conv1.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.conv1.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.conv2.conv.weight - torch.Size([144, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.conv2.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.conv2.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv1.weight - torch.Size([6, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv1.bias - torch.Size([6]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv2.weight - torch.Size([144, 6, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv2.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.conv3.conv.weight - torch.Size([24, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.conv3.bn.weight - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage2.unit2.conv3.bn.bias - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.conv1.conv.weight - torch.Size([144, 24, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.conv1.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.conv1.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.conv2.conv.weight - torch.Size([144, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.conv2.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.conv2.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv1.weight - torch.Size([6, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv1.bias - torch.Size([6]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv2.weight - torch.Size([144, 6, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv2.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.conv3.conv.weight - torch.Size([40, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,921 - mmcv - INFO -
backbone.features.stage3.unit1.conv3.bn.weight - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit1.conv3.bn.bias - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.conv1.conv.weight - torch.Size([240, 40, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.conv1.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.conv1.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.conv2.conv.weight - torch.Size([240, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.conv2.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.conv2.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv1.weight - torch.Size([10, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv1.bias - torch.Size([10]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv2.weight - torch.Size([240, 10, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv2.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.conv3.conv.weight - torch.Size([40, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.conv3.bn.weight - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage3.unit2.conv3.bn.bias - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.conv1.conv.weight - torch.Size([240, 40, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.conv1.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.conv1.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.conv2.conv.weight - torch.Size([240, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.conv2.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.conv2.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv1.weight - torch.Size([10, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv1.bias - torch.Size([10]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv2.weight - torch.Size([240, 10, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv2.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.conv3.conv.weight - torch.Size([80, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.conv3.bn.weight - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit1.conv3.bn.bias - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit2.conv1.conv.weight - torch.Size([480, 80, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit2.conv1.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,922 - mmcv - INFO -
backbone.features.stage4.unit2.conv1.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit2.conv2.conv.weight - torch.Size([480, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit2.conv2.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit2.conv2.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv1.weight - torch.Size([20, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv1.bias - torch.Size([20]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv2.weight - torch.Size([480, 20, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv2.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit2.conv3.conv.weight - torch.Size([80, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit2.conv3.bn.weight - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit2.conv3.bn.bias - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.conv1.conv.weight - torch.Size([480, 80, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.conv1.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.conv1.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.conv2.conv.weight - torch.Size([480, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.conv2.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.conv2.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv1.weight - torch.Size([20, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv1.bias - torch.Size([20]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv2.weight - torch.Size([480, 20, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv2.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.conv3.conv.weight - torch.Size([80, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.conv3.bn.weight - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit3.conv3.bn.bias - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit4.conv1.conv.weight - torch.Size([480, 80, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit4.conv1.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit4.conv1.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit4.conv2.conv.weight - torch.Size([480, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit4.conv2.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit4.conv2.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv1.weight - torch.Size([20, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,923 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv1.bias - torch.Size([20]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv2.weight - torch.Size([480, 20, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv2.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit4.conv3.conv.weight - torch.Size([112, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit4.conv3.bn.weight - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit4.conv3.bn.bias - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.conv1.conv.weight - torch.Size([672, 112, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.conv1.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.conv1.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.conv2.conv.weight - torch.Size([672, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.conv2.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.conv2.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv1.weight - torch.Size([28, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv1.bias - torch.Size([28]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv2.weight - torch.Size([672, 28, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv2.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.conv3.conv.weight - torch.Size([112, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.conv3.bn.weight - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit5.conv3.bn.bias - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.conv1.conv.weight - torch.Size([672, 112, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.conv1.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.conv1.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.conv2.conv.weight - torch.Size([672, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.conv2.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.conv2.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv1.weight - torch.Size([28, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv1.bias - torch.Size([28]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv2.weight - torch.Size([672, 28, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv2.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.conv3.conv.weight - torch.Size([112, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.conv3.bn.weight - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,924 - mmcv - INFO -
backbone.features.stage4.unit6.conv3.bn.bias - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.conv1.conv.weight - torch.Size([672, 112, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.conv1.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.conv1.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.conv2.conv.weight - torch.Size([672, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.conv2.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.conv2.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv1.weight - torch.Size([28, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv1.bias - torch.Size([28]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv2.weight - torch.Size([672, 28, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv2.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.conv3.conv.weight - torch.Size([192, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit1.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.conv2.conv.weight - torch.Size([1152, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.conv3.conv.weight - torch.Size([192, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit2.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit3.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit3.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit3.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,925 - mmcv - INFO -
backbone.features.stage5.unit3.conv2.conv.weight - torch.Size([1152, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit3.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit3.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit3.conv3.conv.weight - torch.Size([192, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit3.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit3.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.conv2.conv.weight - torch.Size([1152, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.conv3.conv.weight - torch.Size([192, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit4.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit5.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit5.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit5.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit5.conv2.conv.weight - torch.Size([1152, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit5.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit5.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,926 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,927 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,927 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,927 - mmcv - INFO -
backbone.features.stage5.unit5.conv3.conv.weight - torch.Size([320, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,927 - mmcv - INFO -
backbone.features.stage5.unit5.conv3.bn.weight - torch.Size([320]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,927 - mmcv - INFO -
backbone.features.stage5.unit5.conv3.bn.bias - torch.Size([320]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,927 - mmcv - INFO -
backbone.features.final_block.conv.weight - torch.Size([1280, 320, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,927 - mmcv - INFO -
backbone.features.final_block.bn.weight - torch.Size([1280]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,927 - mmcv - INFO -
backbone.features.final_block.bn.bias - torch.Size([1280]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-10 18:25:55,927 - mmcv - INFO -
head.fc.weight - torch.Size([102, 1280]):
NormalInit: mean=0, std=0.01, bias=0
2023-07-10 18:25:55,927 - mmcv - INFO -
head.fc.bias - torch.Size([102]):
NormalInit: mean=0, std=0.01, bias=0
2023-07-10 18:25:57,371 | INFO : Task Adaptation: [] => ['BACKGROUND_Google', 'Faces', 'Faces_easy', 'Leopards', 'Motorbikes', 'accordion', 'airplanes', 'anchor', 'ant', 'barrel', 'bass', 'beaver', 'binocular', 'bonsai', 'brain', 'brontosaurus', 'buddha', 'butterfly', 'camera', 'cannon', 'car_side', 'ceiling_fan', 'cellphone', 'chair', 'chandelier', 'cougar_body', 'cougar_face', 'crab', 'crayfish', 'crocodile', 'crocodile_head', 'cup', 'dalmatian', 'dollar_bill', 'dolphin', 'dragonfly', 'electric_guitar', 'elephant', 'emu', 'euphonium', 'ewer', 'ferry', 'flamingo', 'flamingo_head', 'garfield', 'gerenuk', 'gramophone', 'grand_piano', 'hawksbill', 'headphone', 'hedgehog', 'helicopter', 'ibis', 'inline_skate', 'joshua_tree', 'kangaroo', 'ketch', 'lamp', 'laptop', 'llama', 'lobster', 'lotus', 'mandolin', 'mayfly', 'menorah', 'metronome', 'minaret', 'nautilus', 'octopus', 'okapi', 'pagoda', 'panda', 'pigeon', 'pizza', 'platypus', 'pyramid', 'revolver', 'rhino', 'rooster', 'saxophone', 'schooner', 'scissors', 'scorpion', 'sea_horse', 'snoopy', 'soccer_ball', 'stapler', 'starfish', 'stegosaurus', 'stop_sign', 'strawberry', 'sunflower', 'tick', 'trilobite', 'umbrella', 'watch', 'water_lilly', 'wheelchair', 'wild_cat', 'windsor_chair', 'wrench', 'yin_yang']
2023-07-10 18:25:57,371 | INFO : - Efficient Mode: True
2023-07-10 18:25:57,371 | INFO : - Sampler type: balanced
2023-07-10 18:25:57,371 | INFO : - Sampler flag: False
2023-07-10 18:25:57,372 - mmcls - INFO - Start running, host: dwekr@sooah-desktop, work_dir: /home/dwekr/workspace/datum/outputs/logs
2023-07-10 18:25:57,372 - mmcls - INFO - Hooks will be executed in the following order:
before_run:
(VERY_HIGH ) CosineAnnealingLrUpdaterHook
(ABOVE_NORMAL) Fp16SAMOptimizerHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) CheckpointHookWithValResults
(NORMAL ) CancelInterfaceHook
(NORMAL ) AdaptiveTrainSchedulingHook
(NORMAL ) LoggerReplaceHook
(71 ) OTXProgressHook
(75 ) LazyEarlyStoppingHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
--------------------
before_train_epoch:
(VERY_HIGH ) CosineAnnealingLrUpdaterHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) TaskAdaptHook
(LOW ) IterTimerHook
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
(LOWEST ) ForceTrainModeHook
--------------------
before_train_iter:
(VERY_HIGH ) CosineAnnealingLrUpdaterHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) AdaptiveTrainSchedulingHook
(LOW ) IterTimerHook
(71 ) OTXProgressHook
--------------------
after_train_iter:
(ABOVE_NORMAL) Fp16SAMOptimizerHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) CheckpointHookWithValResults
(LOW ) IterTimerHook
(71 ) OTXProgressHook
(75 ) LazyEarlyStoppingHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
--------------------
after_train_epoch:
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) CheckpointHookWithValResults
(71 ) OTXProgressHook
(75 ) LazyEarlyStoppingHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
--------------------
before_val_epoch:
(NORMAL ) TaskAdaptHook
(LOW ) IterTimerHook
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
--------------------
before_val_iter:
(LOW ) IterTimerHook
(71 ) OTXProgressHook
--------------------
after_val_iter:
(LOW ) IterTimerHook
(71 ) OTXProgressHook
--------------------
after_val_epoch:
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
--------------------
after_run:
(NORMAL ) CancelInterfaceHook
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
--------------------
2023-07-10 18:25:57,372 - mmcls - INFO - workflow: [('train', 1)], max: 90 epochs
2023-07-10 18:25:57,373 | INFO : cancel hook is initialized
2023-07-10 18:25:57,373 | INFO : logger in the runner is replaced to the MPA logger
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/torch/utils/tensorboard/__init__.py:4: DeprecationWarning: distutils Version classes are deprecated. Use packaging.version instead.
if not hasattr(tensorboard, "__version__") or LooseVersion(
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/torch/utils/tensorboard/__init__.py:6: DeprecationWarning: distutils Version classes are deprecated. Use packaging.version instead.
) < LooseVersion("1.15"):
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1170.6 task/s, elapsed: 8s, ETA: 0s
2023-07-10 18:26:18,923 | INFO : Epoch [1][100/143] lr: 4.900e-03, eta: 0:29:09, time: 0.137, data_time: 0.004, memory: 3668, current_iters: 99, loss: 1.8926, sharpness: 0.2329, max_loss: 2.1255
2023-07-10 18:26:22,562 | WARNING : training progress 1%
2023-07-10 18:26:24,335 | INFO : Exp name: outputs/logs
2023-07-10 18:26:24,335 | INFO : Epoch [1][143/143] lr: 4.900e-03, eta: 0:39:08, time: 0.127, data_time: 0.000, memory: 3668, current_iters: 142, loss: 0.8262, sharpness: 0.2382, max_loss: 1.0639
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1378.6 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:26:31,004 | INFO : Saving best checkpoint at 1 epochs
2023-07-10 18:26:31,133 | INFO : Exp name: outputs/logs
2023-07-10 18:26:31,133 | INFO : Epoch(val) [1][143] accuracy_top-1: 0.9555, accuracy_top-5: 0.9953, BACKGROUND_Google accuracy: 0.8651, Faces accuracy: 0.9954, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 0.8571, ant accuracy: 0.7381, barrel accuracy: 0.9574, bass accuracy: 0.8889, beaver accuracy: 0.9348, binocular accuracy: 0.9697, bonsai accuracy: 0.9922, brain accuracy: 0.9898, brontosaurus accuracy: 0.7674, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 0.9800, cannon accuracy: 0.9302, car_side accuracy: 1.0000, ceiling_fan accuracy: 0.9149, cellphone accuracy: 1.0000, chair accuracy: 0.8710, chandelier accuracy: 0.9720, cougar_body accuracy: 0.9574, cougar_face accuracy: 0.9420, crab accuracy: 0.9863, crayfish accuracy: 0.9000, crocodile accuracy: 0.9400, crocodile_head accuracy: 0.4510, cup accuracy: 1.0000, dalmatian accuracy: 0.9851, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 0.9200, elephant accuracy: 0.9219, emu accuracy: 0.9623, euphonium accuracy: 0.9844, ewer accuracy: 0.9882, ferry accuracy: 1.0000, flamingo accuracy: 0.9851, flamingo_head accuracy: 0.9778, garfield accuracy: 0.9118, gerenuk accuracy: 0.7941, gramophone accuracy: 1.0000, grand_piano accuracy: 0.9899, hawksbill accuracy: 1.0000, headphone accuracy: 0.9524, hedgehog accuracy: 0.9815, helicopter accuracy: 0.9886, ibis accuracy: 0.9875, inline_skate accuracy: 0.8710, joshua_tree accuracy: 0.9844, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 0.9615, lobster accuracy: 0.5854, lotus accuracy: 0.8939, mandolin accuracy: 0.8140, mayfly accuracy: 0.8750, menorah accuracy: 0.9885, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 0.9455, octopus accuracy: 0.2857, okapi accuracy: 0.9744, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 0.9778, pizza accuracy: 0.9623, platypus accuracy: 0.8529, pyramid accuracy: 1.0000, revolver accuracy: 0.9756, rhino accuracy: 0.9661, rooster accuracy: 0.9388, saxophone accuracy: 1.0000, schooner accuracy: 0.2381, scissors accuracy: 1.0000, scorpion accuracy: 0.9286, sea_horse accuracy: 0.9474, snoopy accuracy: 0.6571, soccer_ball accuracy: 1.0000, stapler accuracy: 0.9333, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 0.9429, sunflower accuracy: 0.9882, tick accuracy: 0.9796, trilobite accuracy: 0.9884, umbrella accuracy: 0.9867, watch accuracy: 0.9958, water_lilly accuracy: 0.5946, wheelchair accuracy: 0.9661, wild_cat accuracy: 0.8235, windsor_chair accuracy: 1.0000, wrench accuracy: 0.8974, yin_yang accuracy: 0.9500, mean accuracy: 0.9293, accuracy: 0.9555, current_iters: 143
2023-07-10 18:26:31,139 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:26:44,023 | INFO : Epoch [2][100/143] lr: 4.899e-03, eta: 0:34:00, time: 0.129, data_time: 0.003, memory: 3668, current_iters: 242, loss: 0.2356, sharpness: 0.1889, max_loss: 0.4245
2023-07-10 18:26:49,454 | INFO : Exp name: outputs/logs
2023-07-10 18:26:49,454 | INFO : Epoch [2][143/143] lr: 4.899e-03, eta: 0:38:04, time: 0.126, data_time: 0.000, memory: 3668, current_iters: 285, loss: 0.2175, sharpness: 0.1879, max_loss: 0.4054
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1342.2 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:26:56,302 | INFO : Saving best checkpoint at 2 epochs
2023-07-10 18:26:56,454 | INFO : Exp name: outputs/logs
2023-07-10 18:26:56,454 | INFO : Epoch(val) [2][143] accuracy_top-1: 0.9843, accuracy_top-5: 0.9996, BACKGROUND_Google accuracy: 0.9422, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 0.9762, ant accuracy: 0.9524, barrel accuracy: 1.0000, bass accuracy: 0.9815, beaver accuracy: 0.9783, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 0.9070, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 0.9535, car_side accuracy: 1.0000, ceiling_fan accuracy: 0.9787, cellphone accuracy: 1.0000, chair accuracy: 0.9839, chandelier accuracy: 0.9813, cougar_body accuracy: 0.9787, cougar_face accuracy: 0.9710, crab accuracy: 0.9589, crayfish accuracy: 0.9714, crocodile accuracy: 0.9400, crocodile_head accuracy: 0.9412, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 0.9333, garfield accuracy: 0.8824, gerenuk accuracy: 0.9706, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 0.9524, hedgehog accuracy: 0.9815, helicopter accuracy: 0.9886, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 0.9688, kangaroo accuracy: 1.0000, ketch accuracy: 0.8947, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.7805, lotus accuracy: 0.9242, mandolin accuracy: 0.9070, mayfly accuracy: 0.9750, menorah accuracy: 0.9885, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 0.8857, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 0.9778, pizza accuracy: 1.0000, platypus accuracy: 0.8824, pyramid accuracy: 0.9825, revolver accuracy: 0.9878, rhino accuracy: 0.9831, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 0.9524, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 0.9825, snoopy accuracy: 0.9714, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 0.9867, watch accuracy: 1.0000, water_lilly accuracy: 0.5135, wheelchair accuracy: 1.0000, wild_cat accuracy: 0.9706, windsor_chair accuracy: 0.9821, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9764, accuracy: 0.9843, current_iters: 286
2023-07-10 18:26:56,459 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:27:09,381 | INFO : Epoch [3][100/143] lr: 4.894e-03, eta: 0:34:56, time: 0.129, data_time: 0.003, memory: 3668, current_iters: 385, loss: 0.1279, sharpness: 0.1522, max_loss: 0.2801
2023-07-10 18:27:14,812 | INFO : Exp name: outputs/logs
2023-07-10 18:27:14,813 | INFO : Epoch [3][143/143] lr: 4.894e-03, eta: 0:37:26, time: 0.126, data_time: 0.000, memory: 3668, current_iters: 428, loss: 0.1352, sharpness: 0.1578, max_loss: 0.2931
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1350.0 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:27:21,623 | INFO : Saving best checkpoint at 3 epochs
2023-07-10 18:27:21,769 | INFO : Exp name: outputs/logs
2023-07-10 18:27:21,769 | INFO : Epoch(val) [3][143] accuracy_top-1: 0.9892, accuracy_top-5: 0.9998, BACKGROUND_Google accuracy: 0.9893, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 0.9762, barrel accuracy: 1.0000, bass accuracy: 0.9630, beaver accuracy: 1.0000, binocular accuracy: 0.9697, bonsai accuracy: 0.9922, brain accuracy: 1.0000, brontosaurus accuracy: 0.9535, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 0.9787, cellphone accuracy: 1.0000, chair accuracy: 0.9194, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 0.9710, crab accuracy: 0.9863, crayfish accuracy: 1.0000, crocodile accuracy: 0.9000, crocodile_head accuracy: 0.9608, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 0.8667, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 0.9706, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.8772, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 0.9872, lobster accuracy: 0.7561, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 0.9885, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 0.9412, pyramid accuracy: 1.0000, revolver accuracy: 0.9878, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 0.9841, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 0.9825, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 0.9831, stop_sign accuracy: 1.0000, strawberry accuracy: 0.9714, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.4324, wheelchair accuracy: 0.9831, wild_cat accuracy: 0.9706, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9827, accuracy: 0.9892, current_iters: 429
2023-07-10 18:27:21,774 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:27:34,766 | INFO : Epoch [4][100/143] lr: 4.887e-03, eta: 0:35:10, time: 0.130, data_time: 0.003, memory: 3668, current_iters: 528, loss: 0.0870, sharpness: 0.1324, max_loss: 0.2194
2023-07-10 18:27:40,221 | INFO : Exp name: outputs/logs
2023-07-10 18:27:40,221 | INFO : Epoch [4][143/143] lr: 4.887e-03, eta: 0:36:58, time: 0.127, data_time: 0.000, memory: 3668, current_iters: 571, loss: 0.0855, sharpness: 0.1296, max_loss: 0.2151
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1329.8 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:27:47,138 | INFO : Saving best checkpoint at 4 epochs
2023-07-10 18:27:47,296 | INFO : Exp name: outputs/logs
2023-07-10 18:27:47,297 | INFO : Epoch(val) [4][143] accuracy_top-1: 0.9929, accuracy_top-5: 0.9999, BACKGROUND_Google accuracy: 0.9893, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 0.9762, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 0.9767, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 0.9787, cellphone accuracy: 1.0000, chair accuracy: 0.9839, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 0.9710, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.7600, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9211, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.7561, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 0.9750, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 0.9714, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 0.9878, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.5946, wheelchair accuracy: 1.0000, wild_cat accuracy: 0.9706, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9883, accuracy: 0.9929, current_iters: 572
2023-07-10 18:27:47,303 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:28:00,299 | INFO : Epoch [5][100/143] lr: 4.876e-03, eta: 0:35:08, time: 0.130, data_time: 0.002, memory: 3668, current_iters: 671, loss: 0.0630, sharpness: 0.1146, max_loss: 0.1776
2023-07-10 18:28:05,805 | INFO : Exp name: outputs/logs
2023-07-10 18:28:05,806 | INFO : Epoch [5][143/143] lr: 4.876e-03, eta: 0:36:32, time: 0.128, data_time: 0.000, memory: 3668, current_iters: 714, loss: 0.0684, sharpness: 0.1209, max_loss: 0.1893
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1331.3 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:28:12,712 | INFO : Saving best checkpoint at 5 epochs
2023-07-10 18:28:12,872 | INFO : Exp name: outputs/logs
2023-07-10 18:28:12,872 | INFO : Epoch(val) [5][143] accuracy_top-1: 0.9942, accuracy_top-5: 0.9998, BACKGROUND_Google accuracy: 0.9936, Faces accuracy: 0.9977, Faces_easy accuracy: 0.9931, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 0.9839, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 0.9855, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.9800, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.8860, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.7805, lotus accuracy: 0.7879, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 0.9841, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 0.8857, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.9459, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9922, accuracy: 0.9942, current_iters: 715
2023-07-10 18:28:12,878 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:28:25,985 | INFO : Epoch [6][100/143] lr: 4.863e-03, eta: 0:35:01, time: 0.131, data_time: 0.003, memory: 3668, current_iters: 814, loss: 0.0465, sharpness: 0.1021, max_loss: 0.1486
2023-07-10 18:28:31,501 | INFO : Exp name: outputs/logs
2023-07-10 18:28:31,501 | INFO : Epoch [6][143/143] lr: 4.863e-03, eta: 0:36:08, time: 0.128, data_time: 0.000, memory: 3668, current_iters: 857, loss: 0.0486, sharpness: 0.1058, max_loss: 0.1545
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1336.2 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:28:38,381 | INFO : Saving best checkpoint at 6 epochs
2023-07-10 18:28:38,533 | INFO : Exp name: outputs/logs
2023-07-10 18:28:38,533 | INFO : Epoch(val) [6][143] accuracy_top-1: 0.9960, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 0.9979, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 0.9855, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.9600, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 0.9851, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9912, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.8293, lotus accuracy: 0.8485, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 0.9714, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 0.8730, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.9730, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9942, accuracy: 0.9960, current_iters: 858
2023-07-10 18:28:38,539 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:28:51,778 | INFO : Epoch [7][100/143] lr: 4.846e-03, eta: 0:34:50, time: 0.132, data_time: 0.003, memory: 3668, current_iters: 957, loss: 0.0439, sharpness: 0.0961, max_loss: 0.1400
2023-07-10 18:28:57,353 | INFO : Exp name: outputs/logs
2023-07-10 18:28:57,354 | INFO : Epoch [7][143/143] lr: 4.846e-03, eta: 0:35:47, time: 0.130, data_time: 0.000, memory: 3668, current_iters: 1000, loss: 0.0388, sharpness: 0.0918, max_loss: 0.1306
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1314.9 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:29:04,348 | INFO : Saving best checkpoint at 7 epochs
2023-07-10 18:29:04,504 | INFO : Exp name: outputs/logs
2023-07-10 18:29:04,504 | INFO : Epoch(val) [7][143] accuracy_top-1: 0.9968, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 0.9979, Faces accuracy: 0.9977, Faces_easy accuracy: 0.9931, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 0.9839, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 0.9855, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.9600, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9561, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.9756, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.6216, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9948, accuracy: 0.9968, current_iters: 1001
2023-07-10 18:29:04,510 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:29:17,693 | INFO : Epoch [8][100/143] lr: 4.827e-03, eta: 0:34:36, time: 0.132, data_time: 0.003, memory: 3668, current_iters: 1100, loss: 0.0333, sharpness: 0.0873, max_loss: 0.1206
2023-07-10 18:29:23,292 | INFO : Exp name: outputs/logs
2023-07-10 18:29:23,292 | INFO : Epoch [8][143/143] lr: 4.827e-03, eta: 0:35:24, time: 0.130, data_time: 0.000, memory: 3668, current_iters: 1143, loss: 0.0333, sharpness: 0.0879, max_loss: 0.1212
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1311.3 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:29:30,300 | INFO : Saving best checkpoint at 8 epochs
2023-07-10 18:29:30,449 | INFO : Exp name: outputs/logs
2023-07-10 18:29:30,449 | INFO : Epoch(val) [8][143] accuracy_top-1: 0.9981, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 0.9855, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.9800, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9737, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.9756, lotus accuracy: 0.9545, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 0.9434, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.9730, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9978, accuracy: 0.9981, current_iters: 1144
2023-07-10 18:29:30,455 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:29:43,771 | INFO : Epoch [9][100/143] lr: 4.805e-03, eta: 0:34:21, time: 0.133, data_time: 0.003, memory: 3668, current_iters: 1243, loss: 0.0331, sharpness: 0.0866, max_loss: 0.1196
2023-07-10 18:29:49,375 | WARNING : training progress 10%
2023-07-10 18:29:49,376 | INFO : Exp name: outputs/logs
2023-07-10 18:29:49,376 | INFO : Epoch [9][143/143] lr: 4.805e-03, eta: 0:35:02, time: 0.131, data_time: 0.000, memory: 3668, current_iters: 1286, loss: 0.0321, sharpness: 0.0835, max_loss: 0.1156
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1320.3 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:29:56,340 | INFO : Saving best checkpoint at 9 epochs
2023-07-10 18:29:56,490 | INFO : Exp name: outputs/logs
2023-07-10 18:29:56,490 | INFO : Epoch(val) [9][143] accuracy_top-1: 0.9986, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.9800, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9825, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.9756, lotus accuracy: 0.9394, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 0.9825, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9985, accuracy: 0.9986, current_iters: 1287
2023-07-10 18:29:56,496 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:30:09,767 | INFO : Epoch [10][100/143] lr: 4.780e-03, eta: 0:34:03, time: 0.133, data_time: 0.003, memory: 3668, current_iters: 1386, loss: 0.0246, sharpness: 0.0816, max_loss: 0.1062
2023-07-10 18:30:15,371 | INFO : Exp name: outputs/logs
2023-07-10 18:30:15,371 | INFO : Epoch [10][143/143] lr: 4.780e-03, eta: 0:34:39, time: 0.130, data_time: 0.000, memory: 3668, current_iters: 1429, loss: 0.0256, sharpness: 0.0801, max_loss: 0.1056
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1318.8 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:30:22,344 | INFO : Saving best checkpoint at 10 epochs
2023-07-10 18:30:22,482 | INFO : Exp name: outputs/logs
2023-07-10 18:30:22,482 | INFO : Epoch(val) [10][143] accuracy_top-1: 0.9988, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 0.9800, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.9800, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 0.9884, ketch accuracy: 0.9912, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.9756, lotus accuracy: 0.9697, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9988, accuracy: 0.9988, current_iters: 1430
2023-07-10 18:30:22,488 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:30:35,822 | INFO : Epoch [11][100/143] lr: 4.752e-03, eta: 0:33:45, time: 0.133, data_time: 0.003, memory: 3668, current_iters: 1529, loss: 0.0211, sharpness: 0.0742, max_loss: 0.0953
2023-07-10 18:30:41,414 | INFO : Exp name: outputs/logs
2023-07-10 18:30:41,414 | INFO : Epoch [11][143/143] lr: 4.752e-03, eta: 0:34:16, time: 0.131, data_time: 0.000, memory: 3668, current_iters: 1572, loss: 0.0230, sharpness: 0.0762, max_loss: 0.0992
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1296.6 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:30:48,503 | INFO : Saving best checkpoint at 11 epochs
2023-07-10 18:30:48,654 | INFO : Exp name: outputs/logs
2023-07-10 18:30:48,654 | INFO : Epoch(val) [11][143] accuracy_top-1: 0.9991, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9825, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 0.9697, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9994, accuracy: 0.9991, current_iters: 1573
2023-07-10 18:30:48,660 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:31:01,987 | INFO : Epoch [12][100/143] lr: 4.722e-03, eta: 0:33:25, time: 0.133, data_time: 0.003, memory: 3668, current_iters: 1672, loss: 0.0193, sharpness: 0.0685, max_loss: 0.0878
2023-07-10 18:31:07,641 | INFO : Exp name: outputs/logs
2023-07-10 18:31:07,641 | INFO : Epoch [12][143/143] lr: 4.722e-03, eta: 0:33:53, time: 0.131, data_time: 0.000, memory: 3668, current_iters: 1715, loss: 0.0197, sharpness: 0.0717, max_loss: 0.0915
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1311.1 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:31:14,723 | INFO : Exp name: outputs/logs
2023-07-10 18:31:14,723 | INFO : Epoch(val) [12][143] accuracy_top-1: 0.9988, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9561, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 0.9697, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9992, accuracy: 0.9988, current_iters: 1716
2023-07-10 18:31:14,729 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:31:28,106 | INFO : Epoch [13][100/143] lr: 4.688e-03, eta: 0:33:05, time: 0.134, data_time: 0.003, memory: 3668, current_iters: 1815, loss: 0.0182, sharpness: 0.0729, max_loss: 0.0911
2023-07-10 18:31:33,745 | INFO : Exp name: outputs/logs
2023-07-10 18:31:33,746 | INFO : Epoch [13][143/143] lr: 4.688e-03, eta: 0:33:29, time: 0.131, data_time: 0.000, memory: 3668, current_iters: 1858, loss: 0.0175, sharpness: 0.0714, max_loss: 0.0890
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1291.1 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:31:40,865 | INFO : Saving best checkpoint at 13 epochs
2023-07-10 18:31:41,009 | INFO : Exp name: outputs/logs
2023-07-10 18:31:41,009 | INFO : Epoch(val) [13][143] accuracy_top-1: 0.9992, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.9800, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 0.9697, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9994, accuracy: 0.9992, current_iters: 1859
2023-07-10 18:31:41,014 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:31:54,354 | INFO : Epoch [14][100/143] lr: 4.652e-03, eta: 0:32:44, time: 0.133, data_time: 0.003, memory: 3668, current_iters: 1958, loss: 0.0161, sharpness: 0.0669, max_loss: 0.0830
2023-07-10 18:31:59,981 | INFO : Exp name: outputs/logs
2023-07-10 18:31:59,981 | INFO : Epoch [14][143/143] lr: 4.652e-03, eta: 0:33:05, time: 0.131, data_time: 0.000, memory: 3668, current_iters: 2001, loss: 0.0157, sharpness: 0.0655, max_loss: 0.0813
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1287.1 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:32:07,122 | INFO : Saving best checkpoint at 14 epochs
2023-07-10 18:32:07,258 | INFO : Exp name: outputs/logs
2023-07-10 18:32:07,258 | INFO : Epoch(val) [14][143] accuracy_top-1: 0.9996, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9999, accuracy: 0.9996, current_iters: 2002
2023-07-10 18:32:07,264 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:32:20,583 | INFO : Epoch [15][100/143] lr: 4.613e-03, eta: 0:32:21, time: 0.133, data_time: 0.003, memory: 3668, current_iters: 2101, loss: 0.0148, sharpness: 0.0600, max_loss: 0.0748
2023-07-10 18:32:26,199 | INFO : Exp name: outputs/logs
2023-07-10 18:32:26,200 | INFO : Epoch [15][143/143] lr: 4.613e-03, eta: 0:32:40, time: 0.131, data_time: 0.000, memory: 3668, current_iters: 2144, loss: 0.0143, sharpness: 0.0609, max_loss: 0.0753
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1302.0 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:32:33,334 | INFO : Exp name: outputs/logs
2023-07-10 18:32:33,334 | INFO : Epoch(val) [15][143] accuracy_top-1: 0.9992, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 0.9697, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 0.9841, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9995, accuracy: 0.9992, current_iters: 2145
2023-07-10 18:32:33,340 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:32:46,782 | INFO : Epoch [16][100/143] lr: 4.572e-03, eta: 0:31:59, time: 0.134, data_time: 0.003, memory: 3668, current_iters: 2244, loss: 0.0135, sharpness: 0.0594, max_loss: 0.0730
2023-07-10 18:32:52,416 | INFO : Exp name: outputs/logs
2023-07-10 18:32:52,416 | INFO : Epoch [16][143/143] lr: 4.572e-03, eta: 0:32:16, time: 0.132, data_time: 0.000, memory: 3668, current_iters: 2287, loss: 0.0139, sharpness: 0.0622, max_loss: 0.0761
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1285.5 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:32:59,656 | INFO : Exp name: outputs/logs
2023-07-10 18:32:59,656 | INFO : Epoch(val) [16][143] accuracy_top-1: 0.9992, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 0.9977, Faces_easy accuracy: 0.9931, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 0.9697, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 0.9821, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9994, accuracy: 0.9992, current_iters: 2288
2023-07-10 18:32:59,663 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:33:13,068 | INFO : Epoch [17][100/143] lr: 4.528e-03, eta: 0:31:36, time: 0.134, data_time: 0.003, memory: 3668, current_iters: 2387, loss: 0.0123, sharpness: 0.0587, max_loss: 0.0710
2023-07-10 18:33:18,743 | INFO : Exp name: outputs/logs
2023-07-10 18:33:18,743 | INFO : Epoch [17][143/143] lr: 4.528e-03, eta: 0:31:52, time: 0.132, data_time: 0.000, memory: 3668, current_iters: 2430, loss: 0.0127, sharpness: 0.0581, max_loss: 0.0708
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1304.0 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:33:25,793 | INFO : Saving best checkpoint at 17 epochs
2023-07-10 18:33:25,945 | INFO : Exp name: outputs/logs
2023-07-10 18:33:25,945 | INFO : Epoch(val) [17][143] accuracy_top-1: 0.9996, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 0.9931, Faces_easy accuracy: 0.9977, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9999, accuracy: 0.9996, current_iters: 2431
2023-07-10 18:33:25,951 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:33:39,372 | INFO : Epoch [18][100/143] lr: 4.481e-03, eta: 0:31:13, time: 0.134, data_time: 0.003, memory: 3668, current_iters: 2530, loss: 0.0110, sharpness: 0.0569, max_loss: 0.0680
2023-07-10 18:33:45,053 | WARNING : training progress 20%
2023-07-10 18:33:45,054 | INFO : Exp name: outputs/logs
2023-07-10 18:33:45,054 | INFO : Epoch [18][143/143] lr: 4.481e-03, eta: 0:31:27, time: 0.132, data_time: 0.000, memory: 3668, current_iters: 2573, loss: 0.0121, sharpness: 0.0599, max_loss: 0.0719
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1295.6 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:33:52,227 | INFO : Exp name: outputs/logs
2023-07-10 18:33:52,227 | INFO : Epoch(val) [18][143] accuracy_top-1: 0.9995, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 0.9855, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9998, accuracy: 0.9995, current_iters: 2574
2023-07-10 18:33:52,233 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:34:05,654 | INFO : Epoch [19][100/143] lr: 4.432e-03, eta: 0:30:50, time: 0.134, data_time: 0.003, memory: 3668, current_iters: 2673, loss: 0.0125, sharpness: 0.0554, max_loss: 0.0679
2023-07-10 18:34:11,362 | INFO : Exp name: outputs/logs
2023-07-10 18:34:11,363 | INFO : Epoch [19][143/143] lr: 4.432e-03, eta: 0:31:02, time: 0.132, data_time: 0.000, memory: 3668, current_iters: 2716, loss: 0.0126, sharpness: 0.0569, max_loss: 0.0696
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1296.8 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:34:18,528 | INFO : Exp name: outputs/logs
2023-07-10 18:34:18,528 | INFO : Epoch(val) [19][143] accuracy_top-1: 0.9993, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 0.9412, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9993, accuracy: 0.9993, current_iters: 2717
2023-07-10 18:34:18,534 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:34:31,987 | INFO : Epoch [20][100/143] lr: 4.381e-03, eta: 0:30:26, time: 0.135, data_time: 0.003, memory: 3668, current_iters: 2816, loss: 0.0096, sharpness: 0.0592, max_loss: 0.0688
2023-07-10 18:34:37,766 | INFO : Exp name: outputs/logs
2023-07-10 18:34:37,766 | INFO : Epoch [20][143/143] lr: 4.381e-03, eta: 0:30:38, time: 0.133, data_time: 0.000, memory: 3668, current_iters: 2859, loss: 0.0099, sharpness: 0.0539, max_loss: 0.0639
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1295.7 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:34:44,863 | INFO : Saving best checkpoint at 20 epochs
2023-07-10 18:34:45,014 | INFO : Exp name: outputs/logs
2023-07-10 18:34:45,014 | INFO : Epoch(val) [20][143] accuracy_top-1: 0.9996, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9999, accuracy: 0.9996, current_iters: 2860
2023-07-10 18:34:45,020 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:34:58,512 | INFO : Epoch [21][100/143] lr: 4.327e-03, eta: 0:30:03, time: 0.135, data_time: 0.003, memory: 3668, current_iters: 2959, loss: 0.0098, sharpness: 0.0531, max_loss: 0.0629
2023-07-10 18:35:04,235 | INFO : Exp name: outputs/logs
2023-07-10 18:35:04,235 | INFO : Epoch [21][143/143] lr: 4.327e-03, eta: 0:30:13, time: 0.133, data_time: 0.000, memory: 3668, current_iters: 3002, loss: 0.0119, sharpness: 0.0539, max_loss: 0.0657
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1285.6 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:35:11,387 | INFO : Saving best checkpoint at 21 epochs
2023-07-10 18:35:11,604 | INFO : Exp name: outputs/logs
2023-07-10 18:35:11,604 | INFO : Epoch(val) [21][143] accuracy_top-1: 0.9996, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9999, accuracy: 0.9996, current_iters: 3003
2023-07-10 18:35:11,609 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:35:25,076 | INFO : Epoch [22][100/143] lr: 4.271e-03, eta: 0:29:39, time: 0.135, data_time: 0.003, memory: 3668, current_iters: 3102, loss: 0.0088, sharpness: 0.0485, max_loss: 0.0572
2023-07-10 18:35:30,824 | INFO : Exp name: outputs/logs
2023-07-10 18:35:30,824 | INFO : Epoch [22][143/143] lr: 4.271e-03, eta: 0:29:48, time: 0.133, data_time: 0.000, memory: 3668, current_iters: 3145, loss: 0.0083, sharpness: 0.0470, max_loss: 0.0553
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 9144/9144, 1277.8 task/s, elapsed: 7s, ETA: 0s
2023-07-10 18:35:38,162 | INFO :
Early Stopping at :21 with best accuracy: 0.9995625305175782
2023-07-10 18:35:38,163 | INFO : Exp name: outputs/logs
2023-07-10 18:35:38,163 | INFO : Epoch(val) [22][143] accuracy_top-1: 0.9993, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9908, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 0.9697, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9996, accuracy: 0.9993, current_iters: 3146
2023-07-10 18:35:38,176 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-10 18:35:39,248 | INFO : called save_model
2023-07-10 18:35:39,356 | INFO : Final model performance: Performance(score: 0.9995625305175782, dashboard: (115 metric groups))
2023-07-10 18:35:39,357 | INFO : train done.
otx train time elapsed: 0:09:51.762842
otx train CLI report has been generated: outputs/cli_report.log
/home/dwekr/miniconda3/envs/datum/lib/python3.10/tempfile.py:833: ResourceWarning: Implicitly cleaning up <TemporaryDirectory '/tmp/img-cache-iqz52wv7'>
_warnings.warn(warn_message, ResourceWarning)
When training with the entire caltech-101 dataset, the best accuracy is 0.9995625305175782
and the training time is 9 minutes and 51 seconds.
[29]:
!otx train EfficientNet-B0 \
--train-data-roots random_result \
--val-data-roots random_result \
-o outputs_random
[*] Workspace Path: otx-workspace-CLASSIFICATION
[*] Load Model Template ID: Custom_Image_Classification_EfficinetNet-B0
[*] Load Model Name: EfficientNet-B0
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/mmcv/__init__.py:20: UserWarning: On January 1, 2023, MMCV will release v2.0.0, in which it will remove components related to the training process and add a data transformation module. In addition, it will rename the package names mmcv to mmcv-lite and mmcv-full to mmcv. See https://github.com/open-mmlab/mmcv/blob/master/docs/en/compatibility.md for more details.
warnings.warn(
2023-07-08 03:58:52,794 | WARNING : Duplicate key is detected among bases [{'model'}]
[*] - Updated: otx-workspace-CLASSIFICATION/model.py
2023-07-08 03:58:52,823 | WARNING : Duplicate key is detected among bases [{'model'}]
[*] - Updated: otx-workspace-CLASSIFICATION/model_multilabel.py
[*] - Updated: otx-workspace-CLASSIFICATION/data_pipeline.py
[*] - Updated: otx-workspace-CLASSIFICATION/deployment.py
[*] - Updated: otx-workspace-CLASSIFICATION/hpo_config.yaml
2023-07-08 03:58:52,923 | WARNING : Duplicate key is detected among bases [{'model'}]
[*] - Updated: otx-workspace-CLASSIFICATION/model_hierarchical.py
[*] - Updated: otx-workspace-CLASSIFICATION/compression_config.json
[*] Update data configuration file to: otx-workspace-CLASSIFICATION/data.yaml
2023-07-08 03:58:53.770452: W tensorflow/compiler/tf2tensorrt/utils/py_utils.cc:38] TF-TRT Warning: Could not find TensorRT
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/openvino/pyopenvino/__init__.py:10: FutureWarning: The module is private and following namespace `pyopenvino` will be removed in the future
warnings.warn(message="The module is private and following namespace " "`pyopenvino` will be removed in the future", category=FutureWarning)
2023-07-08 03:58:55,877 | INFO : Classification mode: multiclass
2023-07-08 03:58:55,877 | INFO : train()
2023-07-08 03:58:55,887 | INFO : Training seed was set to 5 w/ deterministic=False.
2023-07-08 03:58:55,889 | INFO : Try to create a 0 size memory pool.
2023-07-08 03:58:56,669 | INFO : configure!: training=True
2023-07-08 03:58:56,783 | INFO : init weight - https://github.com/osmr/imgclsmob/releases/download/v0.0.364/efficientnet_b0-0752-0e386130.pth.zip
2023-07-08 03:58:56,807 | INFO : 'in_channels' config in model.head is updated from -1 to 1280
2023-07-08 03:58:56,808 | INFO : configure_data()
2023-07-08 03:58:56,808 | INFO : task config!!!!: training=True
2023-07-08 03:58:56,808 | INFO : train!
2023-07-08 03:58:56,808 | INFO : cfg.gpu_ids = range(0, 1), distributed = False
2023-07-08 03:58:56,826 | INFO : Environment info:
------------------------------------------------------------
sys.platform: linux
Python: 3.10.0 (default, Mar 3 2022, 09:58:08) [GCC 7.5.0]
CUDA available: True
GPU 0,1: GeForce RTX 3090
CUDA_HOME: /usr/local/cuda
NVCC: Cuda compilation tools, release 11.1, V11.1.74
GCC: gcc (Ubuntu 7.5.0-3ubuntu1~18.04) 7.5.0
PyTorch: 1.13.1+cu117
PyTorch compiling details: PyTorch built with:
- GCC 9.3
- C++ Version: 201402
- Intel(R) Math Kernel Library Version 2020.0.0 Product Build 20191122 for Intel(R) 64 architecture applications
- Intel(R) MKL-DNN v2.6.0 (Git Hash 52b5f107dd9cf10910aaa19cb47f3abf9b349815)
- OpenMP 201511 (a.k.a. OpenMP 4.5)
- LAPACK is enabled (usually provided by MKL)
- NNPACK is enabled
- CPU capability usage: AVX2
- CUDA Runtime 11.7
- NVCC architecture flags: -gencode;arch=compute_37,code=sm_37;-gencode;arch=compute_50,code=sm_50;-gencode;arch=compute_60,code=sm_60;-gencode;arch=compute_70,code=sm_70;-gencode;arch=compute_75,code=sm_75;-gencode;arch=compute_80,code=sm_80;-gencode;arch=compute_86,code=sm_86
- CuDNN 8.5
- Magma 2.6.1
- Build settings: BLAS_INFO=mkl, BUILD_TYPE=Release, CUDA_VERSION=11.7, CUDNN_VERSION=8.5.0, CXX_COMPILER=/opt/rh/devtoolset-9/root/usr/bin/c++, CXX_FLAGS= -fabi-version=11 -Wno-deprecated -fvisibility-inlines-hidden -DUSE_PTHREADPOOL -fopenmp -DNDEBUG -DUSE_KINETO -DUSE_FBGEMM -DUSE_QNNPACK -DUSE_PYTORCH_QNNPACK -DUSE_XNNPACK -DSYMBOLICATE_MOBILE_DEBUG_HANDLE -DEDGE_PROFILER_USE_KINETO -O2 -fPIC -Wno-narrowing -Wall -Wextra -Werror=return-type -Werror=non-virtual-dtor -Wno-missing-field-initializers -Wno-type-limits -Wno-array-bounds -Wno-unknown-pragmas -Wunused-local-typedefs -Wno-unused-parameter -Wno-unused-function -Wno-unused-result -Wno-strict-overflow -Wno-strict-aliasing -Wno-error=deprecated-declarations -Wno-stringop-overflow -Wno-psabi -Wno-error=pedantic -Wno-error=redundant-decls -Wno-error=old-style-cast -fdiagnostics-color=always -faligned-new -Wno-unused-but-set-variable -Wno-maybe-uninitialized -fno-math-errno -fno-trapping-math -Werror=format -Werror=cast-function-type -Wno-stringop-overflow, LAPACK_INFO=mkl, PERF_WITH_AVX=1, PERF_WITH_AVX2=1, PERF_WITH_AVX512=1, TORCH_VERSION=1.13.1, USE_CUDA=ON, USE_CUDNN=ON, USE_EXCEPTION_PTR=1, USE_GFLAGS=OFF, USE_GLOG=OFF, USE_MKL=ON, USE_MKLDNN=ON, USE_MPI=OFF, USE_NCCL=ON, USE_NNPACK=ON, USE_OPENMP=ON, USE_ROCM=OFF,
TorchVision: 0.14.1+cu117
OpenCV: 4.7.0
MMCV: 1.7.1
MMCV Compiler: GCC 7.5
MMCV CUDA Compiler: 11.1
MMClassification: 0.25.0+c5ac764
------------------------------------------------------------
2023-07-08 03:58:57,061 | INFO : init weight - https://github.com/osmr/imgclsmob/releases/download/v0.0.364/efficientnet_b0-0752-0e386130.pth.zip
2023-07-08 03:58:57,072 - mmcv - INFO - initialize CustomLinearClsHead with init_cfg {'type': 'Normal', 'layer': 'Linear', 'std': 0.01}
2023-07-08 03:58:57,073 - mmcv - INFO -
backbone.features.init_block.conv.conv.weight - torch.Size([32, 3, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,073 - mmcv - INFO -
backbone.features.init_block.conv.bn.weight - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,073 - mmcv - INFO -
backbone.features.init_block.conv.bn.bias - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,073 - mmcv - INFO -
backbone.features.stage1.unit1.dw_conv.conv.weight - torch.Size([32, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,073 - mmcv - INFO -
backbone.features.stage1.unit1.dw_conv.bn.weight - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,073 - mmcv - INFO -
backbone.features.stage1.unit1.dw_conv.bn.bias - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,073 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv1.weight - torch.Size([8, 32, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,073 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv1.bias - torch.Size([8]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,073 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv2.weight - torch.Size([32, 8, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,073 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv2.bias - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage1.unit1.pw_conv.conv.weight - torch.Size([16, 32, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage1.unit1.pw_conv.bn.weight - torch.Size([16]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage1.unit1.pw_conv.bn.bias - torch.Size([16]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.conv1.conv.weight - torch.Size([96, 16, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.conv1.bn.weight - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.conv1.bn.bias - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.conv2.conv.weight - torch.Size([96, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.conv2.bn.weight - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.conv2.bn.bias - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv1.weight - torch.Size([4, 96, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv1.bias - torch.Size([4]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv2.weight - torch.Size([96, 4, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv2.bias - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.conv3.conv.weight - torch.Size([24, 96, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.conv3.bn.weight - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit1.conv3.bn.bias - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit2.conv1.conv.weight - torch.Size([144, 24, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit2.conv1.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit2.conv1.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit2.conv2.conv.weight - torch.Size([144, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit2.conv2.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit2.conv2.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv1.weight - torch.Size([6, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv1.bias - torch.Size([6]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv2.weight - torch.Size([144, 6, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,074 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv2.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage2.unit2.conv3.conv.weight - torch.Size([24, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage2.unit2.conv3.bn.weight - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage2.unit2.conv3.bn.bias - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.conv1.conv.weight - torch.Size([144, 24, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.conv1.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.conv1.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.conv2.conv.weight - torch.Size([144, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.conv2.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.conv2.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv1.weight - torch.Size([6, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv1.bias - torch.Size([6]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv2.weight - torch.Size([144, 6, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv2.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.conv3.conv.weight - torch.Size([40, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.conv3.bn.weight - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit1.conv3.bn.bias - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit2.conv1.conv.weight - torch.Size([240, 40, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit2.conv1.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit2.conv1.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit2.conv2.conv.weight - torch.Size([240, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit2.conv2.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit2.conv2.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv1.weight - torch.Size([10, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv1.bias - torch.Size([10]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv2.weight - torch.Size([240, 10, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,075 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv2.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage3.unit2.conv3.conv.weight - torch.Size([40, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage3.unit2.conv3.bn.weight - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage3.unit2.conv3.bn.bias - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.conv1.conv.weight - torch.Size([240, 40, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.conv1.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.conv1.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.conv2.conv.weight - torch.Size([240, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.conv2.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.conv2.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv1.weight - torch.Size([10, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv1.bias - torch.Size([10]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv2.weight - torch.Size([240, 10, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv2.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.conv3.conv.weight - torch.Size([80, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.conv3.bn.weight - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit1.conv3.bn.bias - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit2.conv1.conv.weight - torch.Size([480, 80, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit2.conv1.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit2.conv1.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit2.conv2.conv.weight - torch.Size([480, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit2.conv2.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit2.conv2.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv1.weight - torch.Size([20, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv1.bias - torch.Size([20]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv2.weight - torch.Size([480, 20, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,076 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv2.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit2.conv3.conv.weight - torch.Size([80, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit2.conv3.bn.weight - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit2.conv3.bn.bias - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.conv1.conv.weight - torch.Size([480, 80, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.conv1.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.conv1.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.conv2.conv.weight - torch.Size([480, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.conv2.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.conv2.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv1.weight - torch.Size([20, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv1.bias - torch.Size([20]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv2.weight - torch.Size([480, 20, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv2.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.conv3.conv.weight - torch.Size([80, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.conv3.bn.weight - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit3.conv3.bn.bias - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit4.conv1.conv.weight - torch.Size([480, 80, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit4.conv1.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit4.conv1.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit4.conv2.conv.weight - torch.Size([480, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit4.conv2.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit4.conv2.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv1.weight - torch.Size([20, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv1.bias - torch.Size([20]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv2.weight - torch.Size([480, 20, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,077 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv2.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit4.conv3.conv.weight - torch.Size([112, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit4.conv3.bn.weight - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit4.conv3.bn.bias - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.conv1.conv.weight - torch.Size([672, 112, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.conv1.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.conv1.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.conv2.conv.weight - torch.Size([672, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.conv2.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.conv2.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv1.weight - torch.Size([28, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv1.bias - torch.Size([28]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv2.weight - torch.Size([672, 28, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv2.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.conv3.conv.weight - torch.Size([112, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.conv3.bn.weight - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit5.conv3.bn.bias - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit6.conv1.conv.weight - torch.Size([672, 112, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit6.conv1.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit6.conv1.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit6.conv2.conv.weight - torch.Size([672, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit6.conv2.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit6.conv2.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv1.weight - torch.Size([28, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv1.bias - torch.Size([28]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv2.weight - torch.Size([672, 28, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv2.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,078 - mmcv - INFO -
backbone.features.stage4.unit6.conv3.conv.weight - torch.Size([112, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage4.unit6.conv3.bn.weight - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage4.unit6.conv3.bn.bias - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.conv1.conv.weight - torch.Size([672, 112, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.conv1.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.conv1.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.conv2.conv.weight - torch.Size([672, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.conv2.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.conv2.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv1.weight - torch.Size([28, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv1.bias - torch.Size([28]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv2.weight - torch.Size([672, 28, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv2.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.conv3.conv.weight - torch.Size([192, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit1.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit2.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit2.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit2.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit2.conv2.conv.weight - torch.Size([1152, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit2.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit2.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,079 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit2.conv3.conv.weight - torch.Size([192, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit2.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit2.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.conv2.conv.weight - torch.Size([1152, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.conv3.conv.weight - torch.Size([192, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit3.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit4.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit4.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit4.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit4.conv2.conv.weight - torch.Size([1152, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit4.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit4.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,080 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit4.conv3.conv.weight - torch.Size([192, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit4.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit4.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.conv2.conv.weight - torch.Size([1152, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.conv3.conv.weight - torch.Size([320, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.conv3.bn.weight - torch.Size([320]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.stage5.unit5.conv3.bn.bias - torch.Size([320]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.final_block.conv.weight - torch.Size([1280, 320, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.final_block.bn.weight - torch.Size([1280]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
backbone.features.final_block.bn.bias - torch.Size([1280]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 03:58:57,081 - mmcv - INFO -
head.fc.weight - torch.Size([102, 1280]):
NormalInit: mean=0, std=0.01, bias=0
2023-07-08 03:58:57,081 - mmcv - INFO -
head.fc.bias - torch.Size([102]):
NormalInit: mean=0, std=0.01, bias=0
2023-07-08 03:58:58,462 | INFO : Task Adaptation: [] => ['BACKGROUND_Google', 'Faces', 'Faces_easy', 'Leopards', 'Motorbikes', 'accordion', 'airplanes', 'anchor', 'ant', 'barrel', 'bass', 'beaver', 'binocular', 'bonsai', 'brain', 'brontosaurus', 'buddha', 'butterfly', 'camera', 'cannon', 'car_side', 'ceiling_fan', 'cellphone', 'chair', 'chandelier', 'cougar_body', 'cougar_face', 'crab', 'crayfish', 'crocodile', 'crocodile_head', 'cup', 'dalmatian', 'dollar_bill', 'dolphin', 'dragonfly', 'electric_guitar', 'elephant', 'emu', 'euphonium', 'ewer', 'ferry', 'flamingo', 'flamingo_head', 'garfield', 'gerenuk', 'gramophone', 'grand_piano', 'hawksbill', 'headphone', 'hedgehog', 'helicopter', 'ibis', 'inline_skate', 'joshua_tree', 'kangaroo', 'ketch', 'lamp', 'laptop', 'llama', 'lobster', 'lotus', 'mandolin', 'mayfly', 'menorah', 'metronome', 'minaret', 'nautilus', 'octopus', 'okapi', 'pagoda', 'panda', 'pigeon', 'pizza', 'platypus', 'pyramid', 'revolver', 'rhino', 'rooster', 'saxophone', 'schooner', 'scissors', 'scorpion', 'sea_horse', 'snoopy', 'soccer_ball', 'stapler', 'starfish', 'stegosaurus', 'stop_sign', 'strawberry', 'sunflower', 'tick', 'trilobite', 'umbrella', 'watch', 'water_lilly', 'wheelchair', 'wild_cat', 'windsor_chair', 'wrench', 'yin_yang']
2023-07-08 03:58:58,463 | INFO : - Efficient Mode: True
2023-07-08 03:58:58,463 | INFO : - Sampler type: balanced
2023-07-08 03:58:58,463 | INFO : - Sampler flag: False
2023-07-08 03:58:58,463 - mmcls - INFO - Start running, host: dwekr@sooah-desktop, work_dir: /home/dwekr/workspace/datum/outputs_random/logs
2023-07-08 03:58:58,463 - mmcls - INFO - Hooks will be executed in the following order:
before_run:
(VERY_HIGH ) CosineAnnealingLrUpdaterHook
(ABOVE_NORMAL) Fp16SAMOptimizerHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) CheckpointHookWithValResults
(NORMAL ) CancelInterfaceHook
(NORMAL ) AdaptiveTrainSchedulingHook
(NORMAL ) LoggerReplaceHook
(71 ) OTXProgressHook
(75 ) LazyEarlyStoppingHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
--------------------
before_train_epoch:
(VERY_HIGH ) CosineAnnealingLrUpdaterHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) TaskAdaptHook
(LOW ) IterTimerHook
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
(LOWEST ) ForceTrainModeHook
--------------------
before_train_iter:
(VERY_HIGH ) CosineAnnealingLrUpdaterHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) AdaptiveTrainSchedulingHook
(LOW ) IterTimerHook
(71 ) OTXProgressHook
--------------------
after_train_iter:
(ABOVE_NORMAL) Fp16SAMOptimizerHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) CheckpointHookWithValResults
(LOW ) IterTimerHook
(71 ) OTXProgressHook
(75 ) LazyEarlyStoppingHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
--------------------
after_train_epoch:
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) CheckpointHookWithValResults
(71 ) OTXProgressHook
(75 ) LazyEarlyStoppingHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
--------------------
before_val_epoch:
(NORMAL ) TaskAdaptHook
(LOW ) IterTimerHook
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
--------------------
before_val_iter:
(LOW ) IterTimerHook
(71 ) OTXProgressHook
--------------------
after_val_iter:
(LOW ) IterTimerHook
(71 ) OTXProgressHook
--------------------
after_val_epoch:
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
--------------------
after_run:
(NORMAL ) CancelInterfaceHook
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
--------------------
2023-07-08 03:58:58,463 - mmcls - INFO - workflow: [('train', 1)], max: 90 epochs
2023-07-08 03:58:58,464 | INFO : cancel hook is initialized
2023-07-08 03:58:58,464 | INFO : logger in the runner is replaced to the MPA logger
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/torch/utils/tensorboard/__init__.py:4: DeprecationWarning: distutils Version classes are deprecated. Use packaging.version instead.
if not hasattr(tensorboard, "__version__") or LooseVersion(
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/torch/utils/tensorboard/__init__.py:6: DeprecationWarning: distutils Version classes are deprecated. Use packaging.version instead.
) < LooseVersion("1.15"):
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1036.6 task/s, elapsed: 4s, ETA: 0s
2023-07-08 03:59:11,588 | WARNING : training progress 1%
2023-07-08 03:59:12,469 | INFO : Exp name: outputs_random/logs
2023-07-08 03:59:12,469 | INFO : Epoch [1][72/72] lr: 4.900e-03, eta: 0:19:42, time: 0.133, data_time: 0.005, memory: 3668, current_iters: 71, loss: 2.3541, sharpness: 0.2387, max_loss: 2.5822
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1374.8 task/s, elapsed: 3s, ETA: 0s
2023-07-08 03:59:15,815 | INFO : Saving best checkpoint at 1 epochs
2023-07-08 03:59:15,948 | INFO : Exp name: outputs_random/logs
2023-07-08 03:59:15,948 | INFO : Epoch(val) [1][72] accuracy_top-1: 0.8633, accuracy_top-5: 0.9748, BACKGROUND_Google accuracy: 0.9352, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 0.0000, ant accuracy: 0.9167, barrel accuracy: 0.9130, bass accuracy: 0.7917, beaver accuracy: 0.8000, binocular accuracy: 0.2500, bonsai accuracy: 0.9672, brain accuracy: 0.8958, brontosaurus accuracy: 0.0000, buddha accuracy: 0.9615, butterfly accuracy: 0.9778, camera accuracy: 1.0000, cannon accuracy: 0.5789, car_side accuracy: 1.0000, ceiling_fan accuracy: 0.9167, cellphone accuracy: 0.9630, chair accuracy: 0.5862, chandelier accuracy: 0.9434, cougar_body accuracy: 0.1818, cougar_face accuracy: 0.9167, crab accuracy: 0.8108, crayfish accuracy: 0.8810, crocodile accuracy: 0.0000, crocodile_head accuracy: 0.8400, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 0.9286, electric_guitar accuracy: 0.9730, elephant accuracy: 0.9744, emu accuracy: 0.2800, euphonium accuracy: 0.9706, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 0.3333, flamingo_head accuracy: 1.0000, garfield accuracy: 0.0000, gerenuk accuracy: 0.0000, gramophone accuracy: 0.5862, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 0.6957, hedgehog accuracy: 1.0000, helicopter accuracy: 0.9375, ibis accuracy: 0.9737, inline_skate accuracy: 0.3529, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9796, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 0.9787, lobster accuracy: 0.0000, lotus accuracy: 0.5588, mandolin accuracy: 0.0500, mayfly accuracy: 0.0435, menorah accuracy: 0.9048, metronome accuracy: 0.3125, minaret accuracy: 1.0000, nautilus accuracy: 0.9600, octopus accuracy: 0.0000, okapi accuracy: 0.0000, pagoda accuracy: 0.9231, panda accuracy: 0.6818, pigeon accuracy: 0.7037, pizza accuracy: 0.9394, platypus accuracy: 0.6111, pyramid accuracy: 0.4074, revolver accuracy: 0.9767, rhino accuracy: 0.5185, rooster accuracy: 0.9615, saxophone accuracy: 0.7222, schooner accuracy: 0.0000, scissors accuracy: 0.6522, scorpion accuracy: 0.9744, sea_horse accuracy: 0.1923, snoopy accuracy: 0.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 0.7692, starfish accuracy: 0.9783, stegosaurus accuracy: 0.9697, stop_sign accuracy: 0.9600, strawberry accuracy: 0.6316, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 0.9744, umbrella accuracy: 0.9412, watch accuracy: 0.9919, water_lilly accuracy: 0.8889, wheelchair accuracy: 0.9032, wild_cat accuracy: 0.3000, windsor_chair accuracy: 1.0000, wrench accuracy: 0.0000, yin_yang accuracy: 0.8000, mean accuracy: 0.7371, accuracy: 0.8633, current_iters: 72
2023-07-08 03:59:15,954 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 03:59:25,430 | INFO : Exp name: outputs_random/logs
2023-07-08 03:59:25,430 | INFO : Epoch [2][72/72] lr: 4.899e-03, eta: 0:19:23, time: 0.132, data_time: 0.004, memory: 3668, current_iters: 143, loss: 0.4784, sharpness: 0.2138, max_loss: 0.6899
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1386.5 task/s, elapsed: 3s, ETA: 0s
2023-07-08 03:59:28,747 | INFO : Saving best checkpoint at 2 epochs
2023-07-08 03:59:28,903 | INFO : Exp name: outputs_random/logs
2023-07-08 03:59:28,903 | INFO : Epoch(val) [2][72] accuracy_top-1: 0.9685, accuracy_top-5: 0.9987, BACKGROUND_Google accuracy: 0.9636, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 0.9500, ant accuracy: 0.9583, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 0.9167, brontosaurus accuracy: 0.7000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 0.7241, chandelier accuracy: 0.9811, cougar_body accuracy: 0.5455, cougar_face accuracy: 1.0000, crab accuracy: 0.8919, crayfish accuracy: 1.0000, crocodile accuracy: 0.3810, crocodile_head accuracy: 0.8800, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 0.9714, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 0.9744, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 0.9744, flamingo_head accuracy: 1.0000, garfield accuracy: 0.9474, gerenuk accuracy: 0.9474, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 0.9130, hedgehog accuracy: 0.9643, helicopter accuracy: 0.9792, ibis accuracy: 1.0000, inline_skate accuracy: 0.9412, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9796, lamp accuracy: 0.9655, laptop accuracy: 1.0000, llama accuracy: 0.9362, lobster accuracy: 0.7500, lotus accuracy: 0.7353, mandolin accuracy: 0.6000, mayfly accuracy: 0.9565, menorah accuracy: 0.9762, metronome accuracy: 0.9375, minaret accuracy: 1.0000, nautilus accuracy: 0.9600, octopus accuracy: 0.2353, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 0.3929, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 0.9231, snoopy accuracy: 0.7778, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 0.9600, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 0.9706, watch accuracy: 0.9919, water_lilly accuracy: 0.9444, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 0.9444, yin_yang accuracy: 0.9600, mean accuracy: 0.9451, accuracy: 0.9685, current_iters: 144
2023-07-08 03:59:28,909 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 03:59:38,225 | INFO : Exp name: outputs_random/logs
2023-07-08 03:59:38,225 | INFO : Epoch [3][72/72] lr: 4.894e-03, eta: 0:19:02, time: 0.129, data_time: 0.004, memory: 3668, current_iters: 215, loss: 0.1965, sharpness: 0.1831, max_loss: 0.3798
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1372.7 task/s, elapsed: 3s, ETA: 0s
2023-07-08 03:59:41,576 | INFO : Saving best checkpoint at 3 epochs
2023-07-08 03:59:41,724 | INFO : Exp name: outputs_random/logs
2023-07-08 03:59:41,724 | INFO : Epoch(val) [3][72] accuracy_top-1: 0.9882, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 0.9838, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 0.9583, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 0.9200, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 0.9500, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.7619, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 0.9643, electric_guitar accuracy: 0.9459, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 0.9744, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 0.9565, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9388, lamp accuracy: 0.9655, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.7000, lotus accuracy: 0.6176, mandolin accuracy: 0.9500, mayfly accuracy: 0.9565, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 0.7647, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 0.8571, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 0.9231, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9812, accuracy: 0.9882, current_iters: 216
2023-07-08 03:59:41,730 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 03:59:51,049 | INFO : Exp name: outputs_random/logs
2023-07-08 03:59:51,049 | INFO : Epoch [4][72/72] lr: 4.887e-03, eta: 0:18:44, time: 0.129, data_time: 0.004, memory: 3668, current_iters: 287, loss: 0.1137, sharpness: 0.1440, max_loss: 0.2576
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1371.4 task/s, elapsed: 3s, ETA: 0s
2023-07-08 03:59:54,402 | INFO : Saving best checkpoint at 4 epochs
2023-07-08 03:59:54,551 | INFO : Exp name: outputs_random/logs
2023-07-08 03:59:54,551 | INFO : Epoch(val) [4][72] accuracy_top-1: 0.9937, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 0.9838, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 0.9762, crocodile accuracy: 1.0000, crocodile_head accuracy: 0.8000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 0.9459, elephant accuracy: 0.9744, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 0.9744, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.7500, lotus accuracy: 0.9118, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 0.8214, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.9444, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9910, accuracy: 0.9937, current_iters: 288
2023-07-08 03:59:54,557 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:00:03,840 | INFO : Exp name: outputs_random/logs
2023-07-08 04:00:03,840 | INFO : Epoch [5][72/72] lr: 4.876e-03, eta: 0:18:28, time: 0.129, data_time: 0.003, memory: 3668, current_iters: 359, loss: 0.0832, sharpness: 0.1344, max_loss: 0.2180
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1373.4 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:00:07,189 | INFO : Saving best checkpoint at 5 epochs
2023-07-08 04:00:07,342 | INFO : Exp name: outputs_random/logs
2023-07-08 04:00:07,342 | INFO : Epoch(val) [5][72] accuracy_top-1: 0.9956, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 0.9500, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 0.9643, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9388, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.9500, lotus accuracy: 0.7059, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 0.9630, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 0.9744, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 0.9500, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9940, accuracy: 0.9956, current_iters: 360
2023-07-08 04:00:07,348 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:00:16,703 | INFO : Exp name: outputs_random/logs
2023-07-08 04:00:16,703 | INFO : Epoch [6][72/72] lr: 4.863e-03, eta: 0:18:14, time: 0.130, data_time: 0.004, memory: 3668, current_iters: 431, loss: 0.0578, sharpness: 0.1157, max_loss: 0.1733
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1362.1 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:00:20,079 | INFO : Saving best checkpoint at 6 epochs
2023-07-08 04:00:20,214 | INFO : Exp name: outputs_random/logs
2023-07-08 04:00:20,214 | INFO : Epoch(val) [6][72] accuracy_top-1: 0.9976, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 0.9744, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.8776, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.9500, lotus accuracy: 0.9412, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9974, accuracy: 0.9976, current_iters: 432
2023-07-08 04:00:20,221 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:00:29,591 | INFO : Exp name: outputs_random/logs
2023-07-08 04:00:29,591 | INFO : Epoch [7][72/72] lr: 4.846e-03, eta: 0:18:01, time: 0.130, data_time: 0.004, memory: 3668, current_iters: 503, loss: 0.0431, sharpness: 0.1033, max_loss: 0.1471
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1363.6 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:00:32,964 | INFO : Saving best checkpoint at 7 epochs
2023-07-08 04:00:33,116 | INFO : Exp name: outputs_random/logs
2023-07-08 04:00:33,116 | INFO : Epoch(val) [7][72] accuracy_top-1: 0.9985, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.9500, lotus accuracy: 0.9118, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 0.9286, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9979, accuracy: 0.9985, current_iters: 504
2023-07-08 04:00:33,122 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:00:42,515 | INFO : Exp name: outputs_random/logs
2023-07-08 04:00:42,515 | INFO : Epoch [8][72/72] lr: 4.827e-03, eta: 0:17:48, time: 0.130, data_time: 0.004, memory: 3668, current_iters: 575, loss: 0.0410, sharpness: 0.1120, max_loss: 0.1532
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1352.4 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:00:45,918 | INFO : Saving best checkpoint at 8 epochs
2023-07-08 04:00:46,067 | INFO : Exp name: outputs_random/logs
2023-07-08 04:00:46,067 | INFO : Epoch(val) [8][72] accuracy_top-1: 0.9996, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.9500, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9995, accuracy: 0.9996, current_iters: 576
2023-07-08 04:00:46,073 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:00:55,460 | WARNING : training progress 10%
2023-07-08 04:00:55,460 | INFO : Exp name: outputs_random/logs
2023-07-08 04:00:55,460 | INFO : Epoch [9][72/72] lr: 4.805e-03, eta: 0:17:35, time: 0.130, data_time: 0.004, memory: 3668, current_iters: 647, loss: 0.0328, sharpness: 0.0913, max_loss: 0.1241
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1334.2 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:00:58,908 | INFO : Saving best checkpoint at 9 epochs
2023-07-08 04:00:59,071 | INFO : Exp name: outputs_random/logs
2023-07-08 04:00:59,071 | INFO : Epoch(val) [9][72] accuracy_top-1: 0.9996, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.9444, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9994, accuracy: 0.9996, current_iters: 648
2023-07-08 04:00:59,077 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:01:08,505 | INFO : Exp name: outputs_random/logs
2023-07-08 04:01:08,505 | INFO : Epoch [10][72/72] lr: 4.780e-03, eta: 0:17:23, time: 0.131, data_time: 0.004, memory: 3668, current_iters: 719, loss: 0.0297, sharpness: 0.0828, max_loss: 0.1124
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1345.5 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:01:11,924 | INFO : Saving best checkpoint at 10 epochs
2023-07-08 04:01:12,067 | INFO : Exp name: outputs_random/logs
2023-07-08 04:01:12,067 | INFO : Epoch(val) [10][72] accuracy_top-1: 0.9996, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 0.9762, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9997, accuracy: 0.9996, current_iters: 720
2023-07-08 04:01:12,073 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:01:21,558 | INFO : Exp name: outputs_random/logs
2023-07-08 04:01:21,558 | INFO : Epoch [11][72/72] lr: 4.752e-03, eta: 0:17:10, time: 0.132, data_time: 0.004, memory: 3668, current_iters: 791, loss: 0.0259, sharpness: 0.0823, max_loss: 0.1085
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1308.0 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:01:25,147 | INFO : Exp name: outputs_random/logs
2023-07-08 04:01:25,148 | INFO : Epoch(val) [11][72] accuracy_top-1: 0.9989, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9184, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9992, accuracy: 0.9989, current_iters: 792
2023-07-08 04:01:25,153 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:01:34,649 | INFO : Exp name: outputs_random/logs
2023-07-08 04:01:34,649 | INFO : Epoch [12][72/72] lr: 4.722e-03, eta: 0:16:58, time: 0.132, data_time: 0.003, memory: 3668, current_iters: 863, loss: 0.0219, sharpness: 0.0751, max_loss: 0.0971
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1329.9 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:01:38,109 | INFO : Saving best checkpoint at 12 epochs
2023-07-08 04:01:38,260 | INFO : Exp name: outputs_random/logs
2023-07-08 04:01:38,260 | INFO : Epoch(val) [12][72] accuracy_top-1: 0.9996, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9796, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9998, accuracy: 0.9996, current_iters: 864
2023-07-08 04:01:38,266 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:01:47,834 | INFO : Exp name: outputs_random/logs
2023-07-08 04:01:47,834 | INFO : Epoch [13][72/72] lr: 4.688e-03, eta: 0:16:47, time: 0.133, data_time: 0.003, memory: 3668, current_iters: 935, loss: 0.0174, sharpness: 0.0714, max_loss: 0.0894
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1340.7 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:01:51,265 | INFO : Saving best checkpoint at 13 epochs
2023-07-08 04:01:51,419 | INFO : Exp name: outputs_random/logs
2023-07-08 04:01:51,419 | INFO : Epoch(val) [13][72] accuracy_top-1: 0.9996, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 0.9706, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9997, accuracy: 0.9996, current_iters: 936
2023-07-08 04:01:51,425 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:02:00,995 | INFO : Exp name: outputs_random/logs
2023-07-08 04:02:00,995 | INFO : Epoch [14][72/72] lr: 4.652e-03, eta: 0:16:35, time: 0.133, data_time: 0.003, memory: 3668, current_iters: 1007, loss: 0.0168, sharpness: 0.0707, max_loss: 0.0875
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1316.6 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:02:04,488 | INFO : Saving best checkpoint at 14 epochs
2023-07-08 04:02:04,636 | INFO : Exp name: outputs_random/logs
2023-07-08 04:02:04,636 | INFO : Epoch(val) [14][72] accuracy_top-1: 0.9996, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 0.9706, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9997, accuracy: 0.9996, current_iters: 1008
2023-07-08 04:02:04,642 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:02:14,402 | INFO : Exp name: outputs_random/logs
2023-07-08 04:02:14,402 | INFO : Epoch [15][72/72] lr: 4.613e-03, eta: 0:16:24, time: 0.136, data_time: 0.004, memory: 3668, current_iters: 1079, loss: 0.0143, sharpness: 0.0672, max_loss: 0.0815
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1322.3 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:02:17,880 | INFO : Saving best checkpoint at 15 epochs
2023-07-08 04:02:18,040 | INFO : Exp name: outputs_random/logs
2023-07-08 04:02:18,040 | INFO : Epoch(val) [15][72] accuracy_top-1: 0.9998, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 0.9998, current_iters: 1080
2023-07-08 04:02:18,046 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:02:27,683 | INFO : Exp name: outputs_random/logs
2023-07-08 04:02:27,683 | INFO : Epoch [16][72/72] lr: 4.572e-03, eta: 0:16:12, time: 0.134, data_time: 0.003, memory: 3668, current_iters: 1151, loss: 0.0135, sharpness: 0.0682, max_loss: 0.0817
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1339.0 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:02:31,116 | INFO : Saving best checkpoint at 16 epochs
2023-07-08 04:02:31,262 | INFO : Exp name: outputs_random/logs
2023-07-08 04:02:31,262 | INFO : Epoch(val) [16][72] accuracy_top-1: 0.9998, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 0.9998, current_iters: 1152
2023-07-08 04:02:31,268 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:02:40,962 | INFO : Exp name: outputs_random/logs
2023-07-08 04:02:40,962 | INFO : Epoch [17][72/72] lr: 4.528e-03, eta: 0:16:00, time: 0.135, data_time: 0.004, memory: 3668, current_iters: 1223, loss: 0.0133, sharpness: 0.0634, max_loss: 0.0767
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1324.7 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:02:44,437 | INFO : Saving best checkpoint at 17 epochs
2023-07-08 04:02:44,604 | INFO : Exp name: outputs_random/logs
2023-07-08 04:02:44,604 | INFO : Epoch(val) [17][72] accuracy_top-1: 0.9998, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 0.9952, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 0.9998, current_iters: 1224
2023-07-08 04:02:44,610 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:02:54,321 | WARNING : training progress 20%
2023-07-08 04:02:54,322 | INFO : Exp name: outputs_random/logs
2023-07-08 04:02:54,322 | INFO : Epoch [18][72/72] lr: 4.481e-03, eta: 0:15:48, time: 0.135, data_time: 0.004, memory: 3668, current_iters: 1295, loss: 0.0124, sharpness: 0.0689, max_loss: 0.0813
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1326.1 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:02:57,790 | INFO : Saving best checkpoint at 18 epochs
2023-07-08 04:02:57,946 | INFO : Exp name: outputs_random/logs
2023-07-08 04:02:57,946 | INFO : Epoch(val) [18][72] accuracy_top-1: 0.9998, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 0.9952, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 0.9998, current_iters: 1296
2023-07-08 04:02:57,952 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:03:07,601 | INFO : Exp name: outputs_random/logs
2023-07-08 04:03:07,601 | INFO : Epoch [19][72/72] lr: 4.432e-03, eta: 0:15:36, time: 0.134, data_time: 0.004, memory: 3668, current_iters: 1367, loss: 0.0088, sharpness: 0.0531, max_loss: 0.0618
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1310.1 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:03:11,113 | INFO : Saving best checkpoint at 19 epochs
2023-07-08 04:03:11,267 | INFO : Exp name: outputs_random/logs
2023-07-08 04:03:11,268 | INFO : Epoch(val) [19][72] accuracy_top-1: 0.9998, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 0.9952, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 0.9998, current_iters: 1368
2023-07-08 04:03:11,274 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:03:20,969 | INFO : Exp name: outputs_random/logs
2023-07-08 04:03:20,970 | INFO : Epoch [20][72/72] lr: 4.381e-03, eta: 0:15:24, time: 0.135, data_time: 0.004, memory: 3668, current_iters: 1439, loss: 0.0110, sharpness: 0.0645, max_loss: 0.0757
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1303.1 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:03:24,500 | INFO : Saving best checkpoint at 20 epochs
2023-07-08 04:03:24,671 | INFO : Exp name: outputs_random/logs
2023-07-08 04:03:24,672 | INFO : Epoch(val) [20][72] accuracy_top-1: 0.9998, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 0.9998, current_iters: 1440
2023-07-08 04:03:24,678 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:03:34,420 | INFO : Exp name: outputs_random/logs
2023-07-08 04:03:34,420 | INFO : Epoch [21][72/72] lr: 4.327e-03, eta: 0:15:12, time: 0.135, data_time: 0.004, memory: 3668, current_iters: 1511, loss: 0.0109, sharpness: 0.0560, max_loss: 0.0670
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1312.5 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:03:37,924 | INFO : Saving best checkpoint at 21 epochs
2023-07-08 04:03:38,082 | INFO : Exp name: outputs_random/logs
2023-07-08 04:03:38,083 | INFO : Epoch(val) [21][72] accuracy_top-1: 0.9998, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 0.9998, current_iters: 1512
2023-07-08 04:03:38,089 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:03:47,847 | INFO : Exp name: outputs_random/logs
2023-07-08 04:03:47,847 | INFO : Epoch [22][72/72] lr: 4.271e-03, eta: 0:14:59, time: 0.136, data_time: 0.004, memory: 3668, current_iters: 1583, loss: 0.0085, sharpness: 0.0544, max_loss: 0.0629
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1303.2 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:03:51,375 | INFO : Saving best checkpoint at 22 epochs
2023-07-08 04:03:51,528 | INFO : Exp name: outputs_random/logs
2023-07-08 04:03:51,528 | INFO : Epoch(val) [22][72] accuracy_top-1: 0.9998, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 0.9998, current_iters: 1584
2023-07-08 04:03:51,534 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:04:01,305 | INFO : Exp name: outputs_random/logs
2023-07-08 04:04:01,305 | INFO : Epoch [23][72/72] lr: 4.212e-03, eta: 0:14:47, time: 0.136, data_time: 0.004, memory: 3668, current_iters: 1655, loss: 0.0080, sharpness: 0.0496, max_loss: 0.0576
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1296.6 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:04:04,852 | INFO : Saving best checkpoint at 23 epochs
2023-07-08 04:04:05,008 | INFO :
Early Stopping at :22 with best accuracy: 0.9997812652587891
2023-07-08 04:04:05,008 | INFO : Exp name: outputs_random/logs
2023-07-08 04:04:05,008 | INFO : Epoch(val) [23][72] accuracy_top-1: 0.9998, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9952, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 0.9998, current_iters: 1656
2023-07-08 04:04:05,014 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:04:06,083 | INFO : called save_model
2023-07-08 04:04:06,187 | INFO : Final model performance: Performance(score: 0.9997812652587891, dashboard: (115 metric groups))
2023-07-08 04:04:06,187 | INFO : train done.
otx train time elapsed: 0:05:16.034499
otx train CLI report has been generated: outputs_random/cli_report.log
/home/dwekr/miniconda3/envs/datum/lib/python3.10/tempfile.py:833: ResourceWarning: Implicitly cleaning up <TemporaryDirectory '/tmp/img-cache-on7bagrf'>
_warnings.warn(warn_message, ResourceWarning)
When pruning the dataset using the random
method with a ratio of 0.5
, the best accuracy achieved is 0.9997812652587891
and the training time is 5 minutes and 16 seconds. There was a 46.55% reduction in time while maintaining similar performance.
[30]:
!otx train EfficientNet-B0 \
--train-data-roots centroid_result \
--val-data-roots centroid_result \
-o output_centroid
[*] Workspace Path: otx-workspace-CLASSIFICATION
[*] Load Model Template ID: Custom_Image_Classification_EfficinetNet-B0
[*] Load Model Name: EfficientNet-B0
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/mmcv/__init__.py:20: UserWarning: On January 1, 2023, MMCV will release v2.0.0, in which it will remove components related to the training process and add a data transformation module. In addition, it will rename the package names mmcv to mmcv-lite and mmcv-full to mmcv. See https://github.com/open-mmlab/mmcv/blob/master/docs/en/compatibility.md for more details.
warnings.warn(
2023-07-08 04:14:17,920 | WARNING : Duplicate key is detected among bases [{'model'}]
[*] - Updated: otx-workspace-CLASSIFICATION/model.py
2023-07-08 04:14:17,949 | WARNING : Duplicate key is detected among bases [{'model'}]
[*] - Updated: otx-workspace-CLASSIFICATION/model_multilabel.py
[*] - Updated: otx-workspace-CLASSIFICATION/data_pipeline.py
[*] - Updated: otx-workspace-CLASSIFICATION/deployment.py
[*] - Updated: otx-workspace-CLASSIFICATION/hpo_config.yaml
2023-07-08 04:14:18,048 | WARNING : Duplicate key is detected among bases [{'model'}]
[*] - Updated: otx-workspace-CLASSIFICATION/model_hierarchical.py
[*] - Updated: otx-workspace-CLASSIFICATION/compression_config.json
[*] Update data configuration file to: otx-workspace-CLASSIFICATION/data.yaml
2023-07-08 04:14:18.894798: W tensorflow/compiler/tf2tensorrt/utils/py_utils.cc:38] TF-TRT Warning: Could not find TensorRT
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/openvino/pyopenvino/__init__.py:10: FutureWarning: The module is private and following namespace `pyopenvino` will be removed in the future
warnings.warn(message="The module is private and following namespace " "`pyopenvino` will be removed in the future", category=FutureWarning)
2023-07-08 04:14:21,041 | INFO : Classification mode: multiclass
2023-07-08 04:14:21,041 | INFO : train()
2023-07-08 04:14:21,052 | INFO : Training seed was set to 5 w/ deterministic=False.
2023-07-08 04:14:21,054 | INFO : Try to create a 0 size memory pool.
2023-07-08 04:14:21,834 | INFO : configure!: training=True
2023-07-08 04:14:21,943 | INFO : init weight - https://github.com/osmr/imgclsmob/releases/download/v0.0.364/efficientnet_b0-0752-0e386130.pth.zip
2023-07-08 04:14:21,969 | INFO : 'in_channels' config in model.head is updated from -1 to 1280
2023-07-08 04:14:21,970 | INFO : configure_data()
2023-07-08 04:14:21,970 | INFO : task config!!!!: training=True
2023-07-08 04:14:21,971 | INFO : train!
2023-07-08 04:14:21,971 | INFO : cfg.gpu_ids = range(0, 1), distributed = False
2023-07-08 04:14:21,989 | INFO : Environment info:
------------------------------------------------------------
sys.platform: linux
Python: 3.10.0 (default, Mar 3 2022, 09:58:08) [GCC 7.5.0]
CUDA available: True
GPU 0,1: GeForce RTX 3090
CUDA_HOME: /usr/local/cuda
NVCC: Cuda compilation tools, release 11.1, V11.1.74
GCC: gcc (Ubuntu 7.5.0-3ubuntu1~18.04) 7.5.0
PyTorch: 1.13.1+cu117
PyTorch compiling details: PyTorch built with:
- GCC 9.3
- C++ Version: 201402
- Intel(R) Math Kernel Library Version 2020.0.0 Product Build 20191122 for Intel(R) 64 architecture applications
- Intel(R) MKL-DNN v2.6.0 (Git Hash 52b5f107dd9cf10910aaa19cb47f3abf9b349815)
- OpenMP 201511 (a.k.a. OpenMP 4.5)
- LAPACK is enabled (usually provided by MKL)
- NNPACK is enabled
- CPU capability usage: AVX2
- CUDA Runtime 11.7
- NVCC architecture flags: -gencode;arch=compute_37,code=sm_37;-gencode;arch=compute_50,code=sm_50;-gencode;arch=compute_60,code=sm_60;-gencode;arch=compute_70,code=sm_70;-gencode;arch=compute_75,code=sm_75;-gencode;arch=compute_80,code=sm_80;-gencode;arch=compute_86,code=sm_86
- CuDNN 8.5
- Magma 2.6.1
- Build settings: BLAS_INFO=mkl, BUILD_TYPE=Release, CUDA_VERSION=11.7, CUDNN_VERSION=8.5.0, CXX_COMPILER=/opt/rh/devtoolset-9/root/usr/bin/c++, CXX_FLAGS= -fabi-version=11 -Wno-deprecated -fvisibility-inlines-hidden -DUSE_PTHREADPOOL -fopenmp -DNDEBUG -DUSE_KINETO -DUSE_FBGEMM -DUSE_QNNPACK -DUSE_PYTORCH_QNNPACK -DUSE_XNNPACK -DSYMBOLICATE_MOBILE_DEBUG_HANDLE -DEDGE_PROFILER_USE_KINETO -O2 -fPIC -Wno-narrowing -Wall -Wextra -Werror=return-type -Werror=non-virtual-dtor -Wno-missing-field-initializers -Wno-type-limits -Wno-array-bounds -Wno-unknown-pragmas -Wunused-local-typedefs -Wno-unused-parameter -Wno-unused-function -Wno-unused-result -Wno-strict-overflow -Wno-strict-aliasing -Wno-error=deprecated-declarations -Wno-stringop-overflow -Wno-psabi -Wno-error=pedantic -Wno-error=redundant-decls -Wno-error=old-style-cast -fdiagnostics-color=always -faligned-new -Wno-unused-but-set-variable -Wno-maybe-uninitialized -fno-math-errno -fno-trapping-math -Werror=format -Werror=cast-function-type -Wno-stringop-overflow, LAPACK_INFO=mkl, PERF_WITH_AVX=1, PERF_WITH_AVX2=1, PERF_WITH_AVX512=1, TORCH_VERSION=1.13.1, USE_CUDA=ON, USE_CUDNN=ON, USE_EXCEPTION_PTR=1, USE_GFLAGS=OFF, USE_GLOG=OFF, USE_MKL=ON, USE_MKLDNN=ON, USE_MPI=OFF, USE_NCCL=ON, USE_NNPACK=ON, USE_OPENMP=ON, USE_ROCM=OFF,
TorchVision: 0.14.1+cu117
OpenCV: 4.7.0
MMCV: 1.7.1
MMCV Compiler: GCC 7.5
MMCV CUDA Compiler: 11.1
MMClassification: 0.25.0+c5ac764
------------------------------------------------------------
2023-07-08 04:14:22,225 | INFO : init weight - https://github.com/osmr/imgclsmob/releases/download/v0.0.364/efficientnet_b0-0752-0e386130.pth.zip
2023-07-08 04:14:22,234 - mmcv - INFO - initialize CustomLinearClsHead with init_cfg {'type': 'Normal', 'layer': 'Linear', 'std': 0.01}
2023-07-08 04:14:22,235 - mmcv - INFO -
backbone.features.init_block.conv.conv.weight - torch.Size([32, 3, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,235 - mmcv - INFO -
backbone.features.init_block.conv.bn.weight - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,235 - mmcv - INFO -
backbone.features.init_block.conv.bn.bias - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,235 - mmcv - INFO -
backbone.features.stage1.unit1.dw_conv.conv.weight - torch.Size([32, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,235 - mmcv - INFO -
backbone.features.stage1.unit1.dw_conv.bn.weight - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,235 - mmcv - INFO -
backbone.features.stage1.unit1.dw_conv.bn.bias - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv1.weight - torch.Size([8, 32, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv1.bias - torch.Size([8]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv2.weight - torch.Size([32, 8, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage1.unit1.se.conv2.bias - torch.Size([32]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage1.unit1.pw_conv.conv.weight - torch.Size([16, 32, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage1.unit1.pw_conv.bn.weight - torch.Size([16]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage1.unit1.pw_conv.bn.bias - torch.Size([16]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.conv1.conv.weight - torch.Size([96, 16, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.conv1.bn.weight - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.conv1.bn.bias - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.conv2.conv.weight - torch.Size([96, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.conv2.bn.weight - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.conv2.bn.bias - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv1.weight - torch.Size([4, 96, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv1.bias - torch.Size([4]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv2.weight - torch.Size([96, 4, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.se.conv2.bias - torch.Size([96]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.conv3.conv.weight - torch.Size([24, 96, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.conv3.bn.weight - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit1.conv3.bn.bias - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit2.conv1.conv.weight - torch.Size([144, 24, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit2.conv1.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit2.conv1.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit2.conv2.conv.weight - torch.Size([144, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit2.conv2.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit2.conv2.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv1.weight - torch.Size([6, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv1.bias - torch.Size([6]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv2.weight - torch.Size([144, 6, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,236 - mmcv - INFO -
backbone.features.stage2.unit2.se.conv2.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage2.unit2.conv3.conv.weight - torch.Size([24, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage2.unit2.conv3.bn.weight - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage2.unit2.conv3.bn.bias - torch.Size([24]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.conv1.conv.weight - torch.Size([144, 24, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.conv1.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.conv1.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.conv2.conv.weight - torch.Size([144, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.conv2.bn.weight - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.conv2.bn.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv1.weight - torch.Size([6, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv1.bias - torch.Size([6]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv2.weight - torch.Size([144, 6, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.se.conv2.bias - torch.Size([144]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.conv3.conv.weight - torch.Size([40, 144, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.conv3.bn.weight - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit1.conv3.bn.bias - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.conv1.conv.weight - torch.Size([240, 40, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.conv1.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.conv1.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.conv2.conv.weight - torch.Size([240, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.conv2.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.conv2.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv1.weight - torch.Size([10, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv1.bias - torch.Size([10]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv2.weight - torch.Size([240, 10, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.se.conv2.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.conv3.conv.weight - torch.Size([40, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.conv3.bn.weight - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage3.unit2.conv3.bn.bias - torch.Size([40]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,237 - mmcv - INFO -
backbone.features.stage4.unit1.conv1.conv.weight - torch.Size([240, 40, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.conv1.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.conv1.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.conv2.conv.weight - torch.Size([240, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.conv2.bn.weight - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.conv2.bn.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv1.weight - torch.Size([10, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv1.bias - torch.Size([10]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv2.weight - torch.Size([240, 10, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.se.conv2.bias - torch.Size([240]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.conv3.conv.weight - torch.Size([80, 240, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.conv3.bn.weight - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit1.conv3.bn.bias - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.conv1.conv.weight - torch.Size([480, 80, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.conv1.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.conv1.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.conv2.conv.weight - torch.Size([480, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.conv2.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.conv2.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv1.weight - torch.Size([20, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv1.bias - torch.Size([20]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv2.weight - torch.Size([480, 20, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.se.conv2.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.conv3.conv.weight - torch.Size([80, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.conv3.bn.weight - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit2.conv3.bn.bias - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit3.conv1.conv.weight - torch.Size([480, 80, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit3.conv1.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit3.conv1.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit3.conv2.conv.weight - torch.Size([480, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit3.conv2.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,238 - mmcv - INFO -
backbone.features.stage4.unit3.conv2.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv1.weight - torch.Size([20, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv1.bias - torch.Size([20]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv2.weight - torch.Size([480, 20, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit3.se.conv2.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit3.conv3.conv.weight - torch.Size([80, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit3.conv3.bn.weight - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit3.conv3.bn.bias - torch.Size([80]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.conv1.conv.weight - torch.Size([480, 80, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.conv1.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.conv1.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.conv2.conv.weight - torch.Size([480, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.conv2.bn.weight - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.conv2.bn.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv1.weight - torch.Size([20, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv1.bias - torch.Size([20]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv2.weight - torch.Size([480, 20, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.se.conv2.bias - torch.Size([480]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.conv3.conv.weight - torch.Size([112, 480, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.conv3.bn.weight - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit4.conv3.bn.bias - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit5.conv1.conv.weight - torch.Size([672, 112, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit5.conv1.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit5.conv1.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit5.conv2.conv.weight - torch.Size([672, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit5.conv2.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit5.conv2.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv1.weight - torch.Size([28, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv1.bias - torch.Size([28]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv2.weight - torch.Size([672, 28, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,239 - mmcv - INFO -
backbone.features.stage4.unit5.se.conv2.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit5.conv3.conv.weight - torch.Size([112, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit5.conv3.bn.weight - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit5.conv3.bn.bias - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.conv1.conv.weight - torch.Size([672, 112, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.conv1.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.conv1.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.conv2.conv.weight - torch.Size([672, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.conv2.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.conv2.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv1.weight - torch.Size([28, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv1.bias - torch.Size([28]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv2.weight - torch.Size([672, 28, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.se.conv2.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.conv3.conv.weight - torch.Size([112, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.conv3.bn.weight - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage4.unit6.conv3.bn.bias - torch.Size([112]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.conv1.conv.weight - torch.Size([672, 112, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.conv1.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.conv1.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.conv2.conv.weight - torch.Size([672, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.conv2.bn.weight - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.conv2.bn.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv1.weight - torch.Size([28, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv1.bias - torch.Size([28]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv2.weight - torch.Size([672, 28, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.se.conv2.bias - torch.Size([672]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.conv3.conv.weight - torch.Size([192, 672, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit1.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit2.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,240 - mmcv - INFO -
backbone.features.stage5.unit2.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit2.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit2.conv2.conv.weight - torch.Size([1152, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit2.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit2.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit2.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit2.conv3.conv.weight - torch.Size([192, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit2.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit2.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.conv2.conv.weight - torch.Size([1152, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.conv3.conv.weight - torch.Size([192, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit3.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit4.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit4.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit4.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit4.conv2.conv.weight - torch.Size([1152, 1, 5, 5]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit4.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,241 - mmcv - INFO -
backbone.features.stage5.unit4.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit4.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit4.conv3.conv.weight - torch.Size([192, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit4.conv3.bn.weight - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit4.conv3.bn.bias - torch.Size([192]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.conv1.conv.weight - torch.Size([1152, 192, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.conv1.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.conv1.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.conv2.conv.weight - torch.Size([1152, 1, 3, 3]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.conv2.bn.weight - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.conv2.bn.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv1.weight - torch.Size([48, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv1.bias - torch.Size([48]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv2.weight - torch.Size([1152, 48, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.se.conv2.bias - torch.Size([1152]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.conv3.conv.weight - torch.Size([320, 1152, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.conv3.bn.weight - torch.Size([320]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.stage5.unit5.conv3.bn.bias - torch.Size([320]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.final_block.conv.weight - torch.Size([1280, 320, 1, 1]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.final_block.bn.weight - torch.Size([1280]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
backbone.features.final_block.bn.bias - torch.Size([1280]):
The value is the same before and after calling `init_weights` of SAMImageClassifier
2023-07-08 04:14:22,242 - mmcv - INFO -
head.fc.weight - torch.Size([102, 1280]):
NormalInit: mean=0, std=0.01, bias=0
2023-07-08 04:14:22,242 - mmcv - INFO -
head.fc.bias - torch.Size([102]):
NormalInit: mean=0, std=0.01, bias=0
2023-07-08 04:14:23,625 | INFO : Task Adaptation: [] => ['BACKGROUND_Google', 'Faces', 'Faces_easy', 'Leopards', 'Motorbikes', 'accordion', 'airplanes', 'anchor', 'ant', 'barrel', 'bass', 'beaver', 'binocular', 'bonsai', 'brain', 'brontosaurus', 'buddha', 'butterfly', 'camera', 'cannon', 'car_side', 'ceiling_fan', 'cellphone', 'chair', 'chandelier', 'cougar_body', 'cougar_face', 'crab', 'crayfish', 'crocodile', 'crocodile_head', 'cup', 'dalmatian', 'dollar_bill', 'dolphin', 'dragonfly', 'electric_guitar', 'elephant', 'emu', 'euphonium', 'ewer', 'ferry', 'flamingo', 'flamingo_head', 'garfield', 'gerenuk', 'gramophone', 'grand_piano', 'hawksbill', 'headphone', 'hedgehog', 'helicopter', 'ibis', 'inline_skate', 'joshua_tree', 'kangaroo', 'ketch', 'lamp', 'laptop', 'llama', 'lobster', 'lotus', 'mandolin', 'mayfly', 'menorah', 'metronome', 'minaret', 'nautilus', 'octopus', 'okapi', 'pagoda', 'panda', 'pigeon', 'pizza', 'platypus', 'pyramid', 'revolver', 'rhino', 'rooster', 'saxophone', 'schooner', 'scissors', 'scorpion', 'sea_horse', 'snoopy', 'soccer_ball', 'stapler', 'starfish', 'stegosaurus', 'stop_sign', 'strawberry', 'sunflower', 'tick', 'trilobite', 'umbrella', 'watch', 'water_lilly', 'wheelchair', 'wild_cat', 'windsor_chair', 'wrench', 'yin_yang']
2023-07-08 04:14:23,625 | INFO : - Efficient Mode: True
2023-07-08 04:14:23,625 | INFO : - Sampler type: balanced
2023-07-08 04:14:23,625 | INFO : - Sampler flag: False
2023-07-08 04:14:23,625 - mmcls - INFO - Start running, host: dwekr@sooah-desktop, work_dir: /home/dwekr/workspace/datum/output_centroid/logs
2023-07-08 04:14:23,626 - mmcls - INFO - Hooks will be executed in the following order:
before_run:
(VERY_HIGH ) CosineAnnealingLrUpdaterHook
(ABOVE_NORMAL) Fp16SAMOptimizerHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) CheckpointHookWithValResults
(NORMAL ) CancelInterfaceHook
(NORMAL ) AdaptiveTrainSchedulingHook
(NORMAL ) LoggerReplaceHook
(71 ) OTXProgressHook
(75 ) LazyEarlyStoppingHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
--------------------
before_train_epoch:
(VERY_HIGH ) CosineAnnealingLrUpdaterHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) TaskAdaptHook
(LOW ) IterTimerHook
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
(LOWEST ) ForceTrainModeHook
--------------------
before_train_iter:
(VERY_HIGH ) CosineAnnealingLrUpdaterHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) AdaptiveTrainSchedulingHook
(LOW ) IterTimerHook
(71 ) OTXProgressHook
--------------------
after_train_iter:
(ABOVE_NORMAL) Fp16SAMOptimizerHook
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) CheckpointHookWithValResults
(LOW ) IterTimerHook
(71 ) OTXProgressHook
(75 ) LazyEarlyStoppingHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
--------------------
after_train_epoch:
(ABOVE_NORMAL) CustomEvalHook
(NORMAL ) CheckpointHookWithValResults
(71 ) OTXProgressHook
(75 ) LazyEarlyStoppingHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
--------------------
before_val_epoch:
(NORMAL ) TaskAdaptHook
(LOW ) IterTimerHook
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
--------------------
before_val_iter:
(LOW ) IterTimerHook
(71 ) OTXProgressHook
--------------------
after_val_iter:
(LOW ) IterTimerHook
(71 ) OTXProgressHook
--------------------
after_val_epoch:
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
(VERY_LOW ) OTXLoggerHook
(VERY_LOW ) MemCacheHook
--------------------
after_run:
(NORMAL ) CancelInterfaceHook
(71 ) OTXProgressHook
(VERY_LOW ) TextLoggerHook
(VERY_LOW ) TensorboardLoggerHook
--------------------
2023-07-08 04:14:23,626 - mmcls - INFO - workflow: [('train', 1)], max: 90 epochs
2023-07-08 04:14:23,626 | INFO : cancel hook is initialized
2023-07-08 04:14:23,626 | INFO : logger in the runner is replaced to the MPA logger
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/torch/utils/tensorboard/__init__.py:4: DeprecationWarning: distutils Version classes are deprecated. Use packaging.version instead.
if not hasattr(tensorboard, "__version__") or LooseVersion(
/home/dwekr/miniconda3/envs/datum/lib/python3.10/site-packages/torch/utils/tensorboard/__init__.py:6: DeprecationWarning: distutils Version classes are deprecated. Use packaging.version instead.
) < LooseVersion("1.15"):
[>>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 999.7 task/s, elapsed: 5s, ETA: 0s
2023-07-08 04:14:36,941 | WARNING : training progress 1%
2023-07-08 04:14:37,821 | INFO : Exp name: output_centroid/logs
2023-07-08 04:14:37,821 | INFO : Epoch [1][72/72] lr: 4.900e-03, eta: 0:19:46, time: 0.133, data_time: 0.005, memory: 3668, current_iters: 71, loss: 2.2499, sharpness: 0.2340, max_loss: 2.4720
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1318.3 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:14:41,309 | INFO : Saving best checkpoint at 1 epochs
2023-07-08 04:14:41,443 | INFO : Exp name: output_centroid/logs
2023-07-08 04:14:41,443 | INFO : Epoch(val) [1][72] accuracy_top-1: 0.8497, accuracy_top-5: 0.9538, BACKGROUND_Google accuracy: 0.8674, Faces accuracy: 0.9930, Faces_easy accuracy: 0.9701, Leopards accuracy: 1.0000, Motorbikes accuracy: 0.9962, accordion accuracy: 0.1667, airplanes accuracy: 0.9967, anchor accuracy: 0.0000, ant accuracy: 0.0000, barrel accuracy: 0.0000, bass accuracy: 0.6786, beaver accuracy: 0.3750, binocular accuracy: 0.7059, bonsai accuracy: 0.9545, brain accuracy: 0.8958, brontosaurus accuracy: 0.0000, buddha accuracy: 1.0000, butterfly accuracy: 0.7333, camera accuracy: 0.9524, cannon accuracy: 0.5882, car_side accuracy: 1.0000, ceiling_fan accuracy: 0.3158, cellphone accuracy: 1.0000, chair accuracy: 0.0000, chandelier accuracy: 0.9706, cougar_body accuracy: 0.0000, cougar_face accuracy: 1.0000, crab accuracy: 0.0000, crayfish accuracy: 0.8222, crocodile accuracy: 0.0909, crocodile_head accuracy: 0.7000, cup accuracy: 0.8261, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 0.8333, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 0.3929, garfield accuracy: 0.0000, gerenuk accuracy: 0.0000, gramophone accuracy: 0.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 0.2500, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 0.9000, inline_skate accuracy: 0.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 0.2000, ketch accuracy: 0.9259, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 0.9630, lobster accuracy: 0.0000, lotus accuracy: 0.0870, mandolin accuracy: 0.7500, mayfly accuracy: 0.0000, menorah accuracy: 0.9492, metronome accuracy: 0.0000, minaret accuracy: 1.0000, nautilus accuracy: 0.9615, octopus accuracy: 0.0000, okapi accuracy: 0.0000, pagoda accuracy: 0.0000, panda accuracy: 0.7917, pigeon accuracy: 0.0000, pizza accuracy: 0.3636, platypus accuracy: 0.8621, pyramid accuracy: 0.9722, revolver accuracy: 0.9722, rhino accuracy: 0.4000, rooster accuracy: 0.0000, saxophone accuracy: 0.0000, schooner accuracy: 0.8393, scissors accuracy: 0.9583, scorpion accuracy: 0.9839, sea_horse accuracy: 0.0000, snoopy accuracy: 0.1579, soccer_ball accuracy: 1.0000, stapler accuracy: 0.0526, starfish accuracy: 1.0000, stegosaurus accuracy: 0.0588, stop_sign accuracy: 1.0000, strawberry accuracy: 0.7931, sunflower accuracy: 0.9744, tick accuracy: 1.0000, trilobite accuracy: 0.9778, umbrella accuracy: 0.9811, watch accuracy: 1.0000, water_lilly accuracy: 0.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 0.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 0.8667, yin_yang accuracy: 0.9487, mean accuracy: 0.6350, accuracy: 0.8497, current_iters: 72
2023-07-08 04:14:41,449 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:14:50,681 | INFO : Exp name: output_centroid/logs
2023-07-08 04:14:50,681 | INFO : Epoch [2][72/72] lr: 4.899e-03, eta: 0:19:10, time: 0.128, data_time: 0.003, memory: 3668, current_iters: 143, loss: 0.5256, sharpness: 0.2175, max_loss: 0.7429
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1331.2 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:14:54,136 | INFO : Saving best checkpoint at 2 epochs
2023-07-08 04:14:54,259 | INFO : Exp name: output_centroid/logs
2023-07-08 04:14:54,259 | INFO : Epoch(val) [2][72] accuracy_top-1: 0.9626, accuracy_top-5: 0.9974, BACKGROUND_Google accuracy: 0.9432, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9701, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 0.9412, ant accuracy: 0.9375, barrel accuracy: 0.8889, bass accuracy: 0.9643, beaver accuracy: 0.5000, binocular accuracy: 1.0000, bonsai accuracy: 0.9773, brain accuracy: 1.0000, brontosaurus accuracy: 0.8421, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 0.9412, car_side accuracy: 1.0000, ceiling_fan accuracy: 0.9474, cellphone accuracy: 1.0000, chair accuracy: 0.9231, chandelier accuracy: 0.9853, cougar_body accuracy: 0.0000, cougar_face accuracy: 1.0000, crab accuracy: 0.9375, crayfish accuracy: 0.9111, crocodile accuracy: 0.7727, crocodile_head accuracy: 1.0000, cup accuracy: 0.9565, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 0.8333, elephant accuracy: 1.0000, emu accuracy: 0.9722, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 0.4286, garfield accuracy: 0.2727, gerenuk accuracy: 0.0000, gramophone accuracy: 0.9412, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 0.8750, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 0.8182, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.8642, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 0.9815, lobster accuracy: 0.7222, lotus accuracy: 0.6522, mandolin accuracy: 0.9643, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 0.9615, octopus accuracy: 0.0769, okapi accuracy: 0.3846, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 0.9231, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 0.9722, rhino accuracy: 1.0000, rooster accuracy: 0.9000, saxophone accuracy: 1.0000, schooner accuracy: 0.9643, scissors accuracy: 1.0000, scorpion accuracy: 0.9677, sea_horse accuracy: 0.9565, snoopy accuracy: 0.8947, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 0.9906, water_lilly accuracy: 0.9231, wheelchair accuracy: 1.0000, wild_cat accuracy: 0.6364, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9139, accuracy: 0.9626, current_iters: 144
2023-07-08 04:14:54,265 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:15:03,562 | INFO : Exp name: output_centroid/logs
2023-07-08 04:15:03,562 | INFO : Epoch [3][72/72] lr: 4.894e-03, eta: 0:18:52, time: 0.129, data_time: 0.003, memory: 3668, current_iters: 215, loss: 0.2172, sharpness: 0.1805, max_loss: 0.3978
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1328.5 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:15:07,024 | INFO : Saving best checkpoint at 3 epochs
2023-07-08 04:15:07,147 | INFO : Exp name: output_centroid/logs
2023-07-08 04:15:07,147 | INFO : Epoch(val) [3][72] accuracy_top-1: 0.9880, accuracy_top-5: 0.9996, BACKGROUND_Google accuracy: 0.9886, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9851, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 0.9984, anchor accuracy: 1.0000, ant accuracy: 0.9375, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 0.9167, binocular accuracy: 1.0000, bonsai accuracy: 0.9773, brain accuracy: 1.0000, brontosaurus accuracy: 0.9474, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 0.9474, cellphone accuracy: 1.0000, chair accuracy: 0.9231, chandelier accuracy: 1.0000, cougar_body accuracy: 0.8000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.7727, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 0.9091, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.8765, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.7778, lotus accuracy: 0.5652, mandolin accuracy: 1.0000, mayfly accuracy: 0.9524, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 0.3846, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9771, accuracy: 0.9880, current_iters: 216
2023-07-08 04:15:07,153 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:15:16,476 | INFO : Exp name: output_centroid/logs
2023-07-08 04:15:16,476 | INFO : Epoch [4][72/72] lr: 4.887e-03, eta: 0:18:38, time: 0.129, data_time: 0.004, memory: 3668, current_iters: 287, loss: 0.1223, sharpness: 0.1482, max_loss: 0.2723
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1328.9 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:15:19,936 | INFO : Saving best checkpoint at 4 epochs
2023-07-08 04:15:20,056 | INFO : Exp name: output_centroid/logs
2023-07-08 04:15:20,056 | INFO : Epoch(val) [4][72] accuracy_top-1: 0.9923, accuracy_top-5: 0.9998, BACKGROUND_Google accuracy: 0.9962, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9776, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 0.9375, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 0.9583, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 0.9778, crocodile accuracy: 0.6364, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 0.9167, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 0.9875, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9877, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.8333, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 0.9231, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 0.8929, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.6154, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9867, accuracy: 0.9923, current_iters: 288
2023-07-08 04:15:20,063 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:15:29,429 | INFO : Exp name: output_centroid/logs
2023-07-08 04:15:29,429 | INFO : Epoch [5][72/72] lr: 4.876e-03, eta: 0:18:25, time: 0.130, data_time: 0.004, memory: 3668, current_iters: 359, loss: 0.0850, sharpness: 0.1300, max_loss: 0.2150
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1323.2 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:15:32,904 | INFO : Saving best checkpoint at 5 epochs
2023-07-08 04:15:33,029 | INFO : Exp name: output_centroid/logs
2023-07-08 04:15:33,029 | INFO : Epoch(val) [5][72] accuracy_top-1: 0.9976, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9925, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 0.9375, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 0.9615, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9630, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.9444, lotus accuracy: 0.9130, mandolin accuracy: 0.9643, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 0.9231, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9961, accuracy: 0.9976, current_iters: 360
2023-07-08 04:15:33,035 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:15:42,458 | INFO : Exp name: output_centroid/logs
2023-07-08 04:15:42,458 | INFO : Epoch [6][72/72] lr: 4.863e-03, eta: 0:18:13, time: 0.131, data_time: 0.004, memory: 3668, current_iters: 431, loss: 0.0607, sharpness: 0.1179, max_loss: 0.1790
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1307.7 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:15:46,032 | INFO : Exp name: output_centroid/logs
2023-07-08 04:15:46,032 | INFO : Epoch(val) [6][72] accuracy_top-1: 0.9963, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9776, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 0.9375, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.9545, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9630, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.8333, lotus accuracy: 1.0000, mandolin accuracy: 0.9643, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 0.9231, okapi accuracy: 1.0000, pagoda accuracy: 0.9375, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.7692, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9927, accuracy: 0.9963, current_iters: 432
2023-07-08 04:15:46,038 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:15:55,469 | INFO : Exp name: output_centroid/logs
2023-07-08 04:15:55,469 | INFO : Epoch [7][72/72] lr: 4.846e-03, eta: 0:18:01, time: 0.131, data_time: 0.003, memory: 3668, current_iters: 503, loss: 0.0472, sharpness: 0.1066, max_loss: 0.1538
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1303.8 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:15:59,056 | INFO : Exp name: output_centroid/logs
2023-07-08 04:15:59,057 | INFO : Epoch(val) [7][72] accuracy_top-1: 0.9974, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9776, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 0.9545, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9383, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.8462, wheelchair accuracy: 1.0000, wild_cat accuracy: 0.9091, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9963, accuracy: 0.9974, current_iters: 504
2023-07-08 04:15:59,062 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:16:08,495 | INFO : Exp name: output_centroid/logs
2023-07-08 04:16:08,495 | INFO : Epoch [8][72/72] lr: 4.827e-03, eta: 0:17:48, time: 0.131, data_time: 0.003, memory: 3668, current_iters: 575, loss: 0.0408, sharpness: 0.1056, max_loss: 0.1471
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1306.6 task/s, elapsed: 3s, ETA: 0s
2023-07-08 04:16:12,014 | INFO : Saving best checkpoint at 8 epochs
2023-07-08 04:16:12,139 | INFO : Exp name: output_centroid/logs
2023-07-08 04:16:12,139 | INFO : Epoch(val) [8][72] accuracy_top-1: 0.9985, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9925, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 0.9444, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 0.9444, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.8462, wheelchair accuracy: 1.0000, wild_cat accuracy: 0.9091, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9964, accuracy: 0.9985, current_iters: 576
2023-07-08 04:16:12,145 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:16:21,603 | WARNING : training progress 10%
2023-07-08 04:16:21,604 | INFO : Exp name: output_centroid/logs
2023-07-08 04:16:21,604 | INFO : Epoch [9][72/72] lr: 4.805e-03, eta: 0:17:36, time: 0.131, data_time: 0.004, memory: 3668, current_iters: 647, loss: 0.0395, sharpness: 0.0994, max_loss: 0.1389
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1293.7 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:16:25,159 | INFO : Saving best checkpoint at 9 epochs
2023-07-08 04:16:25,285 | INFO : Exp name: output_centroid/logs
2023-07-08 04:16:25,285 | INFO : Epoch(val) [9][72] accuracy_top-1: 0.9991, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 0.9851, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 0.8462, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9983, accuracy: 0.9991, current_iters: 648
2023-07-08 04:16:25,291 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:16:34,725 | INFO : Exp name: output_centroid/logs
2023-07-08 04:16:34,725 | INFO : Epoch [10][72/72] lr: 4.780e-03, eta: 0:17:24, time: 0.131, data_time: 0.004, memory: 3668, current_iters: 719, loss: 0.0317, sharpness: 0.0884, max_loss: 0.1215
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1275.3 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:16:38,330 | INFO : Saving best checkpoint at 10 epochs
2023-07-08 04:16:38,454 | INFO : Exp name: output_centroid/logs
2023-07-08 04:16:38,454 | INFO : Epoch(val) [10][72] accuracy_top-1: 0.9996, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 0.9753, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9998, accuracy: 0.9996, current_iters: 720
2023-07-08 04:16:38,460 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:16:47,905 | INFO : Exp name: output_centroid/logs
2023-07-08 04:16:47,905 | INFO : Epoch [11][72/72] lr: 4.752e-03, eta: 0:17:11, time: 0.131, data_time: 0.004, memory: 3668, current_iters: 791, loss: 0.0247, sharpness: 0.0786, max_loss: 0.1035
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1284.3 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:16:51,484 | INFO : Saving best checkpoint at 11 epochs
2023-07-08 04:16:51,605 | INFO : Exp name: output_centroid/logs
2023-07-08 04:16:51,605 | INFO : Epoch(val) [11][72] accuracy_top-1: 1.0000, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 1.0000, current_iters: 792
2023-07-08 04:16:51,611 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:17:01,058 | INFO : Exp name: output_centroid/logs
2023-07-08 04:17:01,058 | INFO : Epoch [12][72/72] lr: 4.722e-03, eta: 0:16:58, time: 0.131, data_time: 0.003, memory: 3668, current_iters: 863, loss: 0.0198, sharpness: 0.0739, max_loss: 0.0936
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1286.1 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:17:04,632 | INFO : Saving best checkpoint at 12 epochs
2023-07-08 04:17:04,759 | INFO : Exp name: output_centroid/logs
2023-07-08 04:17:04,759 | INFO : Epoch(val) [12][72] accuracy_top-1: 1.0000, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 1.0000, current_iters: 864
2023-07-08 04:17:04,765 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:17:14,314 | INFO : Exp name: output_centroid/logs
2023-07-08 04:17:14,314 | INFO : Epoch [13][72/72] lr: 4.688e-03, eta: 0:16:47, time: 0.133, data_time: 0.003, memory: 3668, current_iters: 935, loss: 0.0211, sharpness: 0.0776, max_loss: 0.0986
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1288.6 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:17:17,883 | INFO : Saving best checkpoint at 13 epochs
2023-07-08 04:17:18,011 | INFO : Exp name: output_centroid/logs
2023-07-08 04:17:18,011 | INFO : Epoch(val) [13][72] accuracy_top-1: 1.0000, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 1.0000, current_iters: 936
2023-07-08 04:17:18,017 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:17:27,684 | INFO : Exp name: output_centroid/logs
2023-07-08 04:17:27,684 | INFO : Epoch [14][72/72] lr: 4.652e-03, eta: 0:16:35, time: 0.134, data_time: 0.003, memory: 3668, current_iters: 1007, loss: 0.0185, sharpness: 0.0689, max_loss: 0.0872
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1279.8 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:17:31,340 | INFO : Exp name: output_centroid/logs
2023-07-08 04:17:31,340 | INFO : Epoch(val) [14][72] accuracy_top-1: 0.9998, accuracy_top-5: 0.9998, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 0.9773, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9998, accuracy: 0.9998, current_iters: 1008
2023-07-08 04:17:31,346 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:17:41,053 | INFO : Exp name: output_centroid/logs
2023-07-08 04:17:41,053 | INFO : Epoch [15][72/72] lr: 4.613e-03, eta: 0:16:24, time: 0.135, data_time: 0.004, memory: 3668, current_iters: 1079, loss: 0.0160, sharpness: 0.0755, max_loss: 0.0914
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1297.8 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:17:44,657 | INFO : Exp name: output_centroid/logs
2023-07-08 04:17:44,658 | INFO : Epoch(val) [15][72] accuracy_top-1: 0.9998, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 0.9773, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 0.9998, accuracy: 0.9998, current_iters: 1080
2023-07-08 04:17:44,663 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:17:54,273 | INFO : Exp name: output_centroid/logs
2023-07-08 04:17:54,273 | INFO : Epoch [16][72/72] lr: 4.572e-03, eta: 0:16:12, time: 0.133, data_time: 0.004, memory: 3668, current_iters: 1151, loss: 0.0141, sharpness: 0.0684, max_loss: 0.0826
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1270.9 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:17:57,891 | INFO : Saving best checkpoint at 16 epochs
2023-07-08 04:17:58,021 | INFO : Exp name: output_centroid/logs
2023-07-08 04:17:58,021 | INFO : Epoch(val) [16][72] accuracy_top-1: 1.0000, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 1.0000, current_iters: 1152
2023-07-08 04:17:58,027 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:18:07,665 | INFO : Exp name: output_centroid/logs
2023-07-08 04:18:07,665 | INFO : Epoch [17][72/72] lr: 4.528e-03, eta: 0:16:00, time: 0.134, data_time: 0.004, memory: 3668, current_iters: 1223, loss: 0.0151, sharpness: 0.0687, max_loss: 0.0837
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1251.7 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:18:11,339 | INFO : Saving best checkpoint at 17 epochs
2023-07-08 04:18:11,462 | INFO : Exp name: output_centroid/logs
2023-07-08 04:18:11,462 | INFO : Epoch(val) [17][72] accuracy_top-1: 1.0000, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 1.0000, current_iters: 1224
2023-07-08 04:18:11,468 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:18:21,134 | WARNING : training progress 20%
2023-07-08 04:18:21,134 | INFO : Exp name: output_centroid/logs
2023-07-08 04:18:21,134 | INFO : Epoch [18][72/72] lr: 4.481e-03, eta: 0:15:48, time: 0.134, data_time: 0.003, memory: 3668, current_iters: 1295, loss: 0.0123, sharpness: 0.0603, max_loss: 0.0727
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1260.0 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:18:24,782 | INFO : Saving best checkpoint at 18 epochs
2023-07-08 04:18:24,911 | INFO : Exp name: output_centroid/logs
2023-07-08 04:18:24,911 | INFO : Epoch(val) [18][72] accuracy_top-1: 1.0000, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 1.0000, current_iters: 1296
2023-07-08 04:18:24,917 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:18:34,623 | INFO : Exp name: output_centroid/logs
2023-07-08 04:18:34,624 | INFO : Epoch [19][72/72] lr: 4.432e-03, eta: 0:15:36, time: 0.135, data_time: 0.003, memory: 3668, current_iters: 1367, loss: 0.0105, sharpness: 0.0553, max_loss: 0.0659
[>>>>>>>>>>>>>>>>>>>>>>>>>>] 4572/4572, 1260.4 task/s, elapsed: 4s, ETA: 0s
2023-07-08 04:18:38,272 | INFO : Saving best checkpoint at 19 epochs
2023-07-08 04:18:38,403 | INFO :
Early Stopping at :18 with best accuracy: 1.0
2023-07-08 04:18:38,403 | INFO : Exp name: output_centroid/logs
2023-07-08 04:18:38,403 | INFO : Epoch(val) [19][72] accuracy_top-1: 1.0000, accuracy_top-5: 1.0000, BACKGROUND_Google accuracy: 1.0000, Faces accuracy: 1.0000, Faces_easy accuracy: 1.0000, Leopards accuracy: 1.0000, Motorbikes accuracy: 1.0000, accordion accuracy: 1.0000, airplanes accuracy: 1.0000, anchor accuracy: 1.0000, ant accuracy: 1.0000, barrel accuracy: 1.0000, bass accuracy: 1.0000, beaver accuracy: 1.0000, binocular accuracy: 1.0000, bonsai accuracy: 1.0000, brain accuracy: 1.0000, brontosaurus accuracy: 1.0000, buddha accuracy: 1.0000, butterfly accuracy: 1.0000, camera accuracy: 1.0000, cannon accuracy: 1.0000, car_side accuracy: 1.0000, ceiling_fan accuracy: 1.0000, cellphone accuracy: 1.0000, chair accuracy: 1.0000, chandelier accuracy: 1.0000, cougar_body accuracy: 1.0000, cougar_face accuracy: 1.0000, crab accuracy: 1.0000, crayfish accuracy: 1.0000, crocodile accuracy: 1.0000, crocodile_head accuracy: 1.0000, cup accuracy: 1.0000, dalmatian accuracy: 1.0000, dollar_bill accuracy: 1.0000, dolphin accuracy: 1.0000, dragonfly accuracy: 1.0000, electric_guitar accuracy: 1.0000, elephant accuracy: 1.0000, emu accuracy: 1.0000, euphonium accuracy: 1.0000, ewer accuracy: 1.0000, ferry accuracy: 1.0000, flamingo accuracy: 1.0000, flamingo_head accuracy: 1.0000, garfield accuracy: 1.0000, gerenuk accuracy: 1.0000, gramophone accuracy: 1.0000, grand_piano accuracy: 1.0000, hawksbill accuracy: 1.0000, headphone accuracy: 1.0000, hedgehog accuracy: 1.0000, helicopter accuracy: 1.0000, ibis accuracy: 1.0000, inline_skate accuracy: 1.0000, joshua_tree accuracy: 1.0000, kangaroo accuracy: 1.0000, ketch accuracy: 1.0000, lamp accuracy: 1.0000, laptop accuracy: 1.0000, llama accuracy: 1.0000, lobster accuracy: 1.0000, lotus accuracy: 1.0000, mandolin accuracy: 1.0000, mayfly accuracy: 1.0000, menorah accuracy: 1.0000, metronome accuracy: 1.0000, minaret accuracy: 1.0000, nautilus accuracy: 1.0000, octopus accuracy: 1.0000, okapi accuracy: 1.0000, pagoda accuracy: 1.0000, panda accuracy: 1.0000, pigeon accuracy: 1.0000, pizza accuracy: 1.0000, platypus accuracy: 1.0000, pyramid accuracy: 1.0000, revolver accuracy: 1.0000, rhino accuracy: 1.0000, rooster accuracy: 1.0000, saxophone accuracy: 1.0000, schooner accuracy: 1.0000, scissors accuracy: 1.0000, scorpion accuracy: 1.0000, sea_horse accuracy: 1.0000, snoopy accuracy: 1.0000, soccer_ball accuracy: 1.0000, stapler accuracy: 1.0000, starfish accuracy: 1.0000, stegosaurus accuracy: 1.0000, stop_sign accuracy: 1.0000, strawberry accuracy: 1.0000, sunflower accuracy: 1.0000, tick accuracy: 1.0000, trilobite accuracy: 1.0000, umbrella accuracy: 1.0000, watch accuracy: 1.0000, water_lilly accuracy: 1.0000, wheelchair accuracy: 1.0000, wild_cat accuracy: 1.0000, windsor_chair accuracy: 1.0000, wrench accuracy: 1.0000, yin_yang accuracy: 1.0000, mean accuracy: 1.0000, accuracy: 1.0000, current_iters: 1368
2023-07-08 04:18:38,410 | INFO : MemCacheHandlerBase uses 0 / 0 (0.0%) memory pool and store 0 items.
2023-07-08 04:18:39,479 | INFO : called save_model
2023-07-08 04:18:39,575 | INFO : Final model performance: Performance(score: 1.0, dashboard: (115 metric groups))
2023-07-08 04:18:39,575 | INFO : train done.
otx train time elapsed: 0:04:24.300252
otx train CLI report has been generated: output_centroid/cli_report.log
/home/dwekr/miniconda3/envs/datum/lib/python3.10/tempfile.py:833: ResourceWarning: Implicitly cleaning up <TemporaryDirectory '/tmp/img-cache-yla9hve1'>
_warnings.warn(warn_message, ResourceWarning)
When pruning the dataset using the cluster_random
method with a ratio of 0.5
, the best accuracy achieved is 1.0
and the training time is 4 minutes and 24 seconds. We achieved a time reduction of 46.55% while maintaining similar performance.
When using only 50% of the entire dataset, both random
and cluster_random
methods show performance that is sufficiently good compared to using the entire dataset. Therefore, by creating a representative subset, we can train the model in less time without significant drops in performance.